题目:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。使用python解答
时间: 2024-12-27 22:32:26 浏览: 2
题目描述的是一个简单的字符串分析任务,可以使用Python的内置函数和正则表达式来实现。首先,我们需要遍历整个字符串,然后使用isalpha()、isspace()、isdigit()等函数来检查每个字符的类型,并计数对应类别字符的数量。下面是一个示例Python程序:
```python
def count_chars(s):
alpha_count = sum(1 for c in s if c.isalpha()) # 英文字符
space_count = s.count(' ') # 空格
digit_count = sum(1 for c in s if c.isdigit()) # 数字
other_count = len(s) - alpha_count - space_count - digit_count # 其他字符
return alpha_count, space_count, digit_count, other_count
# 测试函数
input_string = input("请输入一行字符: ")
result = count_chars(input_string)
print(f"英文字母: {result[0]}, 空格: {result[1]}, 数字: {result[2]}, 其它字符: {result[3]}")
相关问题
用python写代码,题目:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数
当然可以,你可以使用Python的内置模块`collections`中的`Counter`来方便地统计字符种类及其出现次数。这里是一个简单的示例:
```python
from collections import Counter
def count_chars(input_string):
# 将字符串转化为小写并分割成单个字符
chars = list(input_string.lower())
# 使用Counter统计每个字符类型的频率
counts = Counter(chars)
# 分别计算字母、空格、数字和其他字符的数量
letters = counts.get('a', 0) + counts.get('z', 0) # 英文字符
spaces = counts[' '] # 空格
digits = sum(1 for c in counts if c.isdigit()) # 数字
others = len(counts) - (letters + spaces + digits) # 其他字符(非字母、空格和数字)
return letters, spaces, digits, others
# 获取用户输入
input_string = input("请输入一行字符: ")
letters, spaces, digits, others = count_chars(input_string)
print(f"字母数量: {letters}")
print(f"空格数量: {spaces}")
print(f"数字数量: {digits}")
print(f"其他字符数量: {others}")
python:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数
你可以使用Python的内置模块`string`来区分不同的字符类型,并结合`collections.Counter`来统计它们的数量。以下是相应的代码:
```python
import string
from collections import Counter
def char_count(input_str):
# 定义字母、数字、空格和其他字符的集合
letters = set(string.ascii_letters)
digits = set(string.digits)
spaces = {chr(32)}
others = set(range(128)) - letters - digits - spaces
# 统计各类字符数量
counts = {
'letters': sum(c in letters for c in input_str),
'digits': sum(c in digits for c in input_str),
'spaces': sum(c in spaces for c in input_str),
'others': sum(c in others for c in input_str)
}
return counts
input_line = input("请输入一行字符: ")
char_counts = char_count(input_line)
for key, value in char_counts.items():
print(f"{key}: {value}")
阅读全文
相关推荐
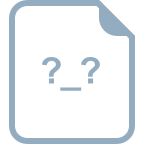
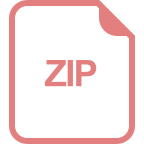













