pytorch MLP代码
时间: 2023-09-24 08:07:21 浏览: 51
以下是使用PyTorch实现多层感知机(MLP)的代码示例:
```python
import torch
import torch.nn as nn
class MLP(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.fc2 = nn.Linear(hidden_size, hidden_size)
self.fc3 = nn.Linear(hidden_size, output_size)
self.relu = nn.ReLU()
def forward(self, x):
x = self.relu(self.fc1(x))
x = self.relu(self.fc2(x))
x = self.fc3(x)
return x
# 定义输入层、隐含层和输出层的维度
input_size = 784
hidden_size = 100
output_size = 10
# 创建MLP模型
model = MLP(input_size, hidden_size, output_size)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# 训练模型
for epoch in range(num_epochs):
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, labels)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失值
if (epoch+1) % 10 == 0:
print(f'Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}')
# 在测试集上评估模型
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
images = images.reshape(-1, 28*28)
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f'Accuracy on the test set: {accuracy:.2f}%')
```
在这个代码示例中,我们首先定义了一个MLP类,该类继承自`nn.Module`,并定义了模型的结构。`nn.Linear`表示全连接层,`nn.ReLU`表示激活函数。在`forward`方法中,我们定义了模型的前向传播过程。
然后,我们定义了输入层、隐含层和输出层的维度,并创建了MLP模型。接下来,我们定义了损失函数和优化器。
在训练过程中,我们使用前向传播计算预测值,并计算损失值。然后,通过反向传播和优化器更新模型的参数。我们还在每个epoch打印损失值。
在测试阶段,我们使用经过训练的模型对测试集进行预测,并计算准确率。
请注意,上述代码只是一个示例,具体的数据准备和训练过程可能有所不同,具体情况需要根据实际需求进行调整。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
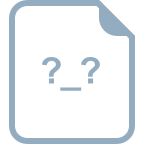
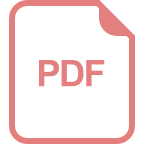














