C++数据存储搜索功能实现
时间: 2024-09-16 16:05:14 浏览: 65
在C++中,数据存储和搜索功能通常通过各种容器和算法来实现。以下是几个常见的方法:
1. **数组**:静态数组是最简单的存储形式,可以直接按索引访问元素。对于范围内的查找,可以遍历数组。
```cpp
int data[10]; // 存储整数
std::size_t index = find(data, data + 10, target); // 查找目标值target
```
2. **动态数组或向量(vector)**:`std::vector`提供了动态大小调整的能力,也支持随机访问和迭代搜索。
```cpp
std::vector<int> vec;
// 存储数据...
auto it = vec.find(target); // 查找指定值
```
3. **关联容器(如map, set, unordered_map, unordered_set)**:这些容器用于基于键(key)查找元素,比如`std::map`使用二叉搜索树。
```cpp
std::map<std::string, int> m;
m["value"] = 42; // 存储键值对
auto it = m.find("value"); // 查找键为"value"的元素
```
4. **线性搜索(如迭代或递归)**:适用于列表或其他无序数据结构,如数组或链表。
```cpp
for (int i = 0; i < list.size(); ++i) {
if (list[i] == target) {
// 找到了
break;
}
}
```
5. **哈希表(如unordered_map)**:提供了常数时间复杂度的平均查找性能,适合大量数据且需要快速检索的情况。
```cpp
std::unordered_map<int, std::string> hash_table;
hash_table[42] = "Value"; // 存储并查找
auto pair = hash_table.find(42);
if (pair != hash_table.end()) {
// 找到了
}
```
阅读全文
相关推荐
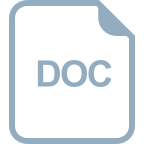
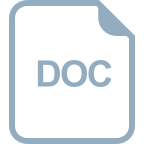
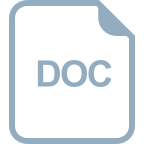
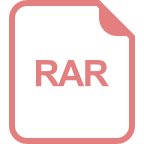
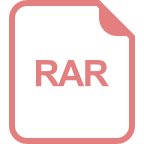
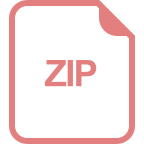
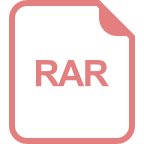
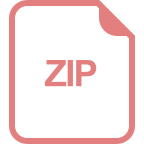
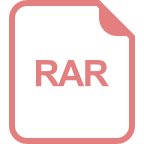
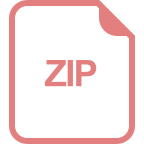
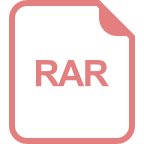
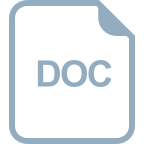
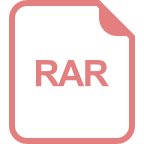
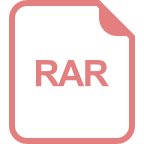
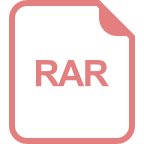
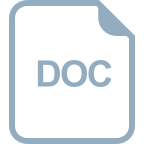
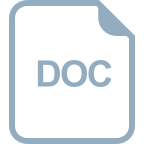
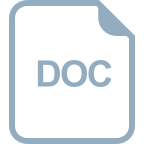
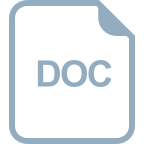