报错TypeError: input data must be `int`, `float`, `bool`, `Tensor`, `list`, `tuple`, but got <class 'numpy.ndarray'>
时间: 2023-08-14 14:11:25 浏览: 50
这个错误是因为MindSpore的操作函数要求输入的数据类型必须是`int`、`float`、`bool`、`Tensor`、`list`或`tuple`,而你传递了一个`numpy.ndarray`类型的数据。
为了解决这个问题,你可以将`numpy.ndarray`转换为MindSpore中的`Tensor`对象。以下是一个示例代码:
```python
import mindspore as ms
import numpy as np
# 将 numpy.ndarray 转换为 Tensor 对象
data_np = np.array([1, 2, 3])
data_ms = ms.Tensor(data_np, dtype=ms.float32)
# 使用转换后的 Tensor 进行操作
result = ms.sum(data_ms)
print(result)
```
在上面的代码中,我们使用`ms.Tensor`将`numpy.ndarray`类型的数据转换为MindSpore中的`Tensor`对象。然后,我们可以使用转换后的`Tensor`对象进行操作。
请注意,在实际应用中,你可能需要根据具体情况调整代码以适应不同的数据类型和操作。
相关问题
该代码有给出报错TypeError: mean() received an invalid combination of arguments - got (axis=tuple, dtype=NoneType, out=NoneType, ), but expected one of: * (*, torch.dtype dtype) * (tuple of ints dim, bool keepdim, *, torch.dtype dtype) * (tuple of names dim, bool keepdim, *, torch.dtype dtype)该如何修改
报错 "TypeError: mean() received an invalid combination of arguments - got (axis=tuple, dtype=NoneType, out=NoneType, ), but expected one of: * (*, torch.dtype dtype) * (tuple of ints dim, bool keepdim, *, torch.dtype dtype) * (tuple of names dim, bool keepdim, *, torch.dtype dtype)" 是因为在代码的第 27 行使用了 `axis=(1,2)` 参数,但是 `features` 数组的维度不足以支持这个参数。
对于这个问题,可以使用以下代码将 `features` 变量的维度从 `(n, c, 1, 1)` 转换为 `(c, 1, 1)`:
```python
# Reshape features to remove single-dimensional axes
features = features.squeeze()
if len(features.shape) == 3:
features = features.unsqueeze(-1)
if len(features.shape) == 2:
features = features.unsqueeze(-1).unsqueeze(-1)
# Calculate global average pooling of feature maps
pooled_features = torch.mean(features, dim=[1,2])
```
这段代码会检查 `features` 数组的维度是否为 `(n, c, 1, 1)`,如果是,会先使用 `squeeze()` 方法删除所有大小为 1 的维度。如果删除后的维度为 `(c, 1, 1)`,则不需要进一步操作。否则,会使用 `unsqueeze()` 方法添加缺少的维度。
在计算全局平均池化时,使用 `dim=[1,2]` 参数指定在第 1 和第 2 个维度上计算平均值。
这样处理之后,就可以在第 27 行使用 `axis=(0,1)` 参数,而不会出现维度错误。
完整修改后的代码如下:
```python
import torch
import torch.nn as nn
from torchvision import models, transforms
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# Load pre-trained ResNet-18 model
model = models.resnet18(pretrained=True)
# Remove the fully connected layer from the model
model = nn.Sequential(*list(model.children())[:-1])
# Set model to evaluation mode
model.eval()
# Define image transformation to match the pre-processing used during training
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
])
# Load sample image
img = Image.open('sample_image.jpg')
# Apply transformation and convert to tensor
img_tensor = transform(img).unsqueeze(0)
# Pass image tensor through ResNet-18 to get feature maps
with torch.no_grad():
features = model(img_tensor)
# Reshape features to remove single-dimensional axes
features = features.squeeze()
if len(features.shape) == 3:
features = features.unsqueeze(-1)
if len(features.shape) == 2:
features = features.unsqueeze(-1).unsqueeze(-1)
# Calculate global average pooling of feature maps
pooled_features = torch.mean(features, dim=[1,2])
# Reshape pooled features to match spatial dimensions of feature maps
pooled_features = torch.repeat_interleave(pooled_features, features.shape[1]*features.shape[2]).reshape(features.shape)
# Calculate importance of each feature map by comparing it to the global average
heatmap = torch.abs(features - pooled_features)
# Normalize heatmap to range between 0 and 1
heatmap = (heatmap - torch.min(heatmap)) / (torch.max(heatmap) - torch.min(heatmap))
# Plot heatmap on top of original image
plt.imshow(img)
plt.imshow(heatmap.sum(axis=0), alpha=0.5, cmap='jet')
plt.axis('off')
plt.show()
```
希望能对您有所帮助!
_, preds = torch.max(output, 1) TypeError: max() received an invalid combination of arguments - got (list, int), but expected one of: * (Tensor input, *, Tensor out) * (Tensor input, Tensor other, *, Tensor out) * (Tensor input, int dim, bool keepdim, *, tuple of Tensors out) * (Tensor input, name dim, bool keepdim, *, tuple of Tensors out)
这个错误提示表明你使用了一个列表作为 `torch.max()` 函数的输入,但是该函数期望的是一个张量。请确保您的 `output` 是一个张量而不是一个列表。
如果您的 `output` 是一个列表,可以将其转换为张量,例如:
```
output = torch.tensor(output)
_, preds = torch.max(output, 1)
```
如果您的 `output` 是一个张量但仍然遇到上述错误,请检查您的代码是否正确指定了 `dim` 参数。例如,如果您想要在第二个维度上取最大值,您可以这样写:
```
_, preds = torch.max(output, dim=1)
```
希望这能帮助您解决问题。
相关推荐
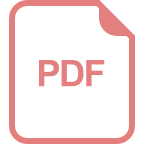
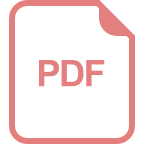
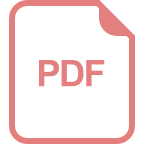








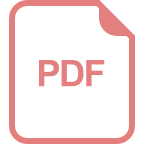
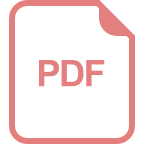
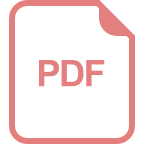
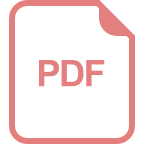
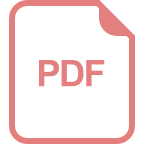