TypeError: mean() received an invalid combination of arguments - got (axis=int, dtype=NoneType, out=NoneType, ), but expected one of: * (*, torch.dtype dtype) * (tuple of ints dim, bool keepdim, *, torch.dtype dtype) * (tuple of names dim, bool keepdim
时间: 2024-03-01 12:53:13 浏览: 388
这个错误是因为你在 `mean()` 函数中使用了 `axis` 参数,但是 `axis` 参数在 PyTorch 1.9 及之前的版本中是不支持的。如果你想在指定维度上计算 tensor 的平均值,可以使用 `dim` 参数来代替 `axis` 参数。示例代码如下:
```python
import torch
# 创建一个 PyTorch tensor
tensor = torch.tensor([[1., 2.], [3., 4.]])
# 沿着第一个维度计算 tensor 的平均值
mean = tensor.mean(dim=0)
# 打印结果
print(mean)
```
输出结果如下:
```
tensor([2., 3.])
```
在 PyTorch 1.10 中,`mean()` 函数已经支持了 `axis` 参数,你可以使用以下代码来计算 tensor 的平均值:
```python
import torch
# 创建一个 PyTorch tensor
tensor = torch.tensor([[1., 2.], [3., 4.]])
# 沿着第一个维度计算 tensor 的平均值
mean = tensor.mean(axis=0)
# 打印结果
print(mean)
```
输出结果与上面的示例代码相同。
相关问题
TypeError: sum() received an invalid combination of arguments - got (out=NoneType, axis=NoneType, ), but expected one of:
To fix the "TypeError: sum() received an invalid combination of arguments" error, you need to provide valid arguments to the sum() function. The sum() function in Python can be used to sum up the elements of an iterable, such as a list or tuple.
The error message suggests that you are using invalid arguments for the sum() function. The sum() function expects either a single iterable argument or two arguments (iterable and start). Here are some examples of correct usages:
1. Summing up elements of a list:
```
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
```
2. Summing up elements of a tuple:
```
numbers = (1, 2, 3, 4, 5)
total = sum(numbers)
```
3. Summing up elements of a list starting from a specific value:
```
numbers = [1, 2, 3, 4, 5]
total = sum(numbers, 10) # Start summing from 10
```
Make sure you are passing a valid iterable as the first argument to the sum() function. If you provide any additional arguments, ensure they are valid as well.
TypeError: max() received an invalid combination of arguments - got (out=NoneType, axis=NoneType, ), but expected one of: * ()
这个错误通常是因为您在调用 `max()` 函数时使用了无效的参数组合。根据错误消息,您传递给 `max()` 函数的参数应该是 `out` 和 `axis`,但是它们的类型被判定为 `NoneType`,这是无效的。
请确保您正确地使用了 `max()` 函数,并传递了有效的参数组合。根据您的需求,您可以在调用 `max()` 函数时提供合适的参数,例如:
```python
maximum = max(iterable) # 获取可迭代对象中的最大值
maximum = max(iterable, default=default_value) # 设置默认值,在可迭代对象为空时返回
maximum = max(iterable, key=key_function) # 根据键函数返回的值来比较并获取最大值
maximum = max(arg1, arg2, ..., argn) # 获取多个参数中的最大值
```
请根据您的具体情况检查并修正调用 `max()` 函数的代码。如果仍然遇到问题,请提供更多的代码和错误信息,以便我能够更好地帮助您解决问题。
阅读全文
相关推荐
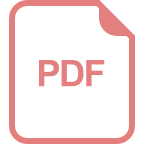
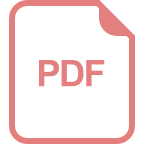
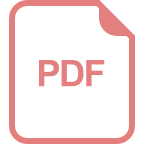













