使用python找到打开一个文本文件并将相同字符的名字替换
时间: 2024-10-25 13:12:05 浏览: 10
在Python中,你可以使用内置的文件操作和字符串处理功能来遍历文本文件,查找指定的字符(这里假设为字符串),然后替换它们。下面是一个简单的例子,展示如何打开、读取、替换并在新的文件中保存修改后的内容:
```python
# 定义要替换的源文件名和目标文件名
source_file = 'input.txt'
target_file = 'output.txt'
# 定义要替换的字符和新的字符
old_char = '名字'
new_char = '123'
# 打开源文件并读取内容
with open(source_file, 'r') as f:
lines = f.readlines()
# 遍历每一行,替换字符
modified_lines = [line.replace(old_char, new_char) for line in lines]
# 写入到新文件
with open(target_file, 'w') as f:
f.writelines(modified_lines)
print(f"替换已完成,新文件保存为 {target_file}.")
#
相关问题
python读取任意一个文本文件 统计其中行数 字符数和单词个数代码
在Python中,你可以使用内置的`open()`函数打开文本文件,并配合`read()`、`split()`等方法来统计行数、字符数和单词个数。这里是一个简单的示例:
```python
def file_stats(filename):
with open(filename, 'r', encoding='utf-8') as f: # 使用'with'确保文件在操作后会被关闭
lines = f.readlines() # 读取所有行
total_chars = sum(len(line) for line in lines) # 计算总字符数
words = len(lines) # 总行数就是单词数,因为每行通常包含一个完整的词
# 对于计算单词数,我们假设每个非空格字符都是一个单词的分隔点
word_count = len(' '.join(lines).split()) # 使用空格合并所有行并计算分隔后的单词数
return lines, total_chars, words, word_count
filename = 'your_file.txt' # 替换为你想要分析的文件名
lines, chars, words, word_count = file_stats(filename)
print(f"行数:{words}, 字符数:{chars}, 单词数:{word_count}")
使用python处理一个文本文件,并将三组字符串的名字分别修改为1,2,3,并将修改后的文件另存为另外一个文本文件
在Python中,你可以使用内置的`open()`函数读取文本文件,然后使用列表推导式或者遍历的方式替换需要更改的部分。这里是一个简单的例子,假设我们有一个名为`input_file.txt`的文件,其中包含三组待替换的字符串:
```python
# 打开原始文件并读取内容
with open('input_file.txt', 'r') as f_in:
file_content = f_in.read()
# 定义替换规则,如将'原始名字1', '原始名字2', '原始名字3'替换为'1', '2', '3'
replacement_dict = {'原始名字1': '1', '原始名字2': '2', '原始名字3': '3'}
# 替换字符串
new_content = file_content.replace(*replacement_dict.items())
# 创建新文件名
output_file_name = 'output_file.txt'
# 将新内容写入到新的文本文件
with open(output_file_name, 'w') as f_out:
f_out.write(new_content)
阅读全文
相关推荐
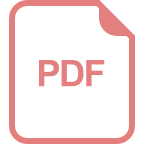
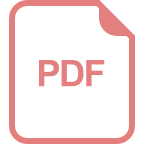
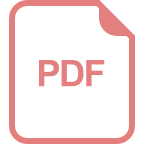













