public class PageSupport { //当前页码-来自于用户输入 private int currentPageNo = 1; //总数量(表) private int totalCount = 0; //页面容量 private int pageSize = 0; //总页数-totalCount/pageSize(+1) private int totalPageCount = 1; public int getCurrentPageNo() { return currentPageNo; } public void setCurrentPageNo(int currentPageNo) { if(currentPageNo > 0){ this.currentPageNo = currentPageNo; } } public int getTotalCount() { return totalCount; } public void setTotalCount(int totalCount) { if(totalCount > 0){ this.totalCount = totalCount; //设置总页数 this.setTotalPageCountByRs(); } } public int getPageSize() { return pageSize; } public void setPageSize(int pageSize) { if(pageSize > 0){ this.pageSize = pageSize; } } public int getTotalPageCount() { return totalPageCount; } public void setTotalPageCount(int totalPageCount) { this.totalPageCount = totalPageCount; } public void setTotalPageCountByRs(){ if(this.totalCount % this.pageSize == 0){ this.totalPageCount = this.totalCount / this.pageSize; }else if(this.totalCount % this.pageSize > 0){ this.totalPageCount = this.totalCount / this.pageSize + 1; }else{ this.totalPageCount = 0; } } }
时间: 2024-04-18 08:32:31 浏览: 19
这段代码定义了一个名为PageSupport的分页支持类,用于管理分页相关的信息。
具体解释如下:
1. currentPageNo:表示当前页码,默认为1。
2. totalCount:表示总记录数量。
3. pageSize:表示每页显示的记录数量。
4. totalPageCount:表示总页数。
该类提供了一些公共的getter和setter方法来获取和设置这些属性的值。其中,setCurrentPageNo和setTotalCount方法中有一些逻辑判断,确保currentPageNo和totalCount的值符合要求。
另外,该类还提供了一个setTotalPageCountByRs方法,用于根据totalCount和pageSize计算并设置totalPageCount的值。根据totalCount和pageSize的关系,计算出totalPageCount的值,并进行相应的赋值。
这个分页支持类可以在应用程序中用于管理分页相关的信息,方便进行分页查询和展示。
相关问题
private Integer pageSize = 10; //每页显示条数 private Integer currentPage; //当前页 private Integer totalPage; //总页数 private Integer totalCount; //总条数 private List<T> dataList;//分页数据使用这个实体类写一个springboot的分页查询
好的,以下是一个简单的Spring Boot分页查询的示例代码:
1. 首先,定义一个分页查询的实体类,例如PageRequest:
```
public class PageRequest {
private int pageSize; //每页显示条数
private int currentPage; //当前页
private int totalPage; //总页数
private int totalCount; //总条数
//getter和setter方法省略
}
```
2. 在Controller中编写分页查询的接口:
```
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public PageRequest getUsers(@RequestParam(defaultValue = "1") Integer page) {
int pageSize = 10; //每页显示10条数据
PageRequest pageRequest = userService.findUsers(page, pageSize); //调用Service层方法查询数据
return pageRequest;
}
}
```
3. 在Service层中实现分页查询的业务逻辑:
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRepository userRepository;
@Override
public PageRequest findUsers(int currentPage, int pageSize) {
PageRequest pageRequest = new PageRequest();
pageRequest.setCurrentPage(currentPage);
pageRequest.setPageSize(pageSize);
int totalCount = userRepository.count(); //查询总条数
pageRequest.setTotalCount(totalCount);
int totalPage = (int) Math.ceil((double) totalCount / pageSize); //计算总页数
pageRequest.setTotalPage(totalPage);
List<User> users = userRepository.findUsers((currentPage - 1) * pageSize, pageSize); //分页查询数据
pageRequest.setDataList(users);
return pageRequest;
}
}
```
4. 在Repository中定义分页查询的SQL语句:
```
@Repository
public class UserRepositoryImpl implements UserRepository {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public int count() {
String sql = "SELECT COUNT(*) FROM user";
return jdbcTemplate.queryForObject(sql, Integer.class);
}
@Override
public List<User> findUsers(int offset, int limit) {
String sql = "SELECT * FROM user LIMIT ?, ?";
return jdbcTemplate.query(sql, new Object[]{offset, limit}, new BeanPropertyRowMapper<>(User.class));
}
}
```
注意事项:
- 分页查询的SQL语句中需要使用LIMIT关键字进行分页查询,LIMIT后面的第一个参数表示查询的起始位置,第二个参数表示查询的条数。
- 在Controller中,我们使用@RequestParam注解来获取当前页码,默认值为1。
- 在Repository中,我们使用JdbcTemplate来执行SQL语句,需要在配置文件中配置数据源和JdbcTemplate的Bean。
教我写一个新手看的懂的分页工具类,语言是java,要求注释详细,当前页用pageNo,每页条数用pageSize
好的,这是一个简单易懂的分页工具类,注释非常详细,可以帮助新手学习使用。代码如下:
```
/**
* 分页工具类
*/
public class PageUtils<T> {
// 当前页码,默认为1
private int pageNo = 1;
// 每页显示的记录数,默认为10
private int pageSize = 10;
// 总记录数
private int totalCount;
// 总页数
private int totalPage;
// 当前页的数据集合
private List<T> list;
/**
* 构造方法,需要传入当前页码和每页显示的记录数
*/
public PageUtils(int pageNo, int pageSize) {
this.pageNo = pageNo;
this.pageSize = pageSize;
}
/**
* 获取当前页码
*/
public int getPageNo() {
return pageNo;
}
/**
* 设置当前页码
*/
public void setPageNo(int pageNo) {
this.pageNo = pageNo;
}
/**
* 获取每页记录数
*/
public int getPageSize() {
return pageSize;
}
/**
* 设置每页记录数
*/
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
/**
* 获取总记录数
*/
public int getTotalCount() {
return totalCount;
}
/**
* 设置总记录数,并计算总页数
*/
public void setTotalCount(int totalCount) {
this.totalCount = totalCount;
this.totalPage = (int) Math.ceil((double) totalCount / pageSize);
}
/**
* 获取总页数
*/
public int getTotalPage() {
return totalPage;
}
/**
* 获取当前页的数据集合
*/
public List<T> getList() {
return list;
}
/**
* 设置当前页的数据集合
*/
public void setList(List<T> list) {
this.list = list;
}
/**
* 获取起始记录的索引值
*/
public int getStartIndex() {
return (pageNo - 1) * pageSize;
}
/**
* 获取结束记录的索引值
*/
public int getEndIndex() {
return pageNo * pageSize;
}
/**
* 是否有上一页
*/
public boolean hasPrevious() {
return pageNo > 1;
}
/**
* 上一页页码
*/
public int getPreviousPage() {
return hasPrevious() ? pageNo - 1 : pageNo;
}
/**
* 是否有下一页
*/
public boolean hasNext() {
return pageNo < totalPage;
}
/**
* 下一页页码
*/
public int getNextPage() {
return hasNext() ? pageNo + 1 : pageNo;
}
}
```
使用方法:
1. 创建分页工具类对象,需要传入当前页码和每页显示的记录数。
```java
PageUtils<User> pageUtils = new PageUtils<>(pageNo, pageSize);
```
2. 设置总记录数和当前页的数据集合。
```java
pageUtils.setTotalCount(totalCount);
pageUtils.setList(list);
```
3. 获取分页信息。
```java
int totalPage = pageUtils.getTotalPage(); // 总页数
int startIndex = pageUtils.getStartIndex(); // 起始记录的索引值
int endIndex = pageUtils.getEndIndex(); // 结束记录的索引值
boolean hasPrevious = pageUtils.hasPrevious(); // 是否有上一页
boolean hasNext = pageUtils.hasNext(); // 是否有下一页
int previousPage = pageUtils.getPreviousPage(); // 上一页页码
int nextPage = pageUtils.getNextPage(); // 下一页页码
List<User> userList = pageUtils.getList(); // 当前页的数据集合
```
希望对你有帮助!
相关推荐
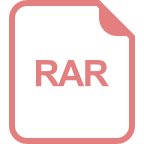
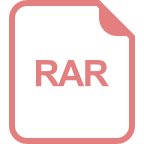
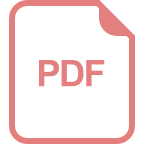












