flutter 使用http 发送post
时间: 2024-10-19 21:03:54 浏览: 18
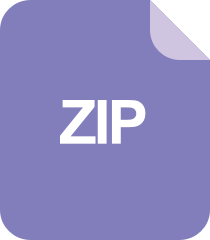
flutter 常用插件整理附加使用实例
在 Flutter 中使用 http 库发送 POST 请求非常直观,你可以按照以下步骤操作:
1. 首先,需要导入 `http` 库:
```dart
import 'package:http/http.dart' as http;
```
2. 创建一个函数,该函数接受你需要发送的数据作为参数:
```dart
Future<http.Response> sendHttpPost(String url, Map<String, dynamic> data) async {
final response = await http.post(
Uri.parse(url),
body: json.encode(data), // 将数据编码成 JSON 格式
headers: { 'Content-Type': 'application/json' }, // 设置 Content-Type 为 JSON
);
return response;
}
```
3. 调用这个函数,传入你的 URL 和需要发送的数据(通常是键值对形式):
```dart
final String apiUrl = "https://your-api-url.com/endpoint";
final Map<String, String> postData = {'key1': 'value1', 'key2': 'value2'};
try {
final response = await sendHttpPost(apiUrl, postData);
// 处理成功响应
} on ClientException catch (e) {
// 捕获可能出现的客户端错误,如网络连接问题
print("发生客户端错误: $e");
} on ServerException catch (e) {
// 捕获服务器端错误,如4XX或5XX状态码
print("发生服务器错误: $e");
} on DioError catch (dioError) {
// 如果使用 Dio 库,按上面的方式处理 DioError
}
```
4. 根据 `response.statusCode` 判断请求是否成功,处理返回的数据(如有必要的话)。
注意,上述示例假设你的 API 接口支持 JSON 格式的请求头。如果需要其他格式(如 form-data),则应调整 `body` 的创建方法。
阅读全文
相关推荐
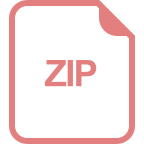
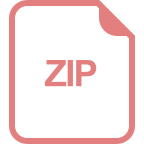





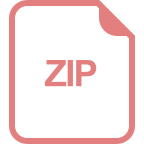








