实现一个C++ property map类
时间: 2024-10-02 12:03:26 浏览: 35
在C++中,我们可以创建一个简单的Property Map类,它通常被用来存储键值对,类似于JavaScript中的Object。这个类可以包含一个私有map容器,提供查找、添加和删除键值对的功能。下面是一个基础版的Property Map实现示例:
```cpp
#include <string>
#include <unordered_map>
class PropertyMap {
public:
// 构造函数
PropertyMap() {}
// 添加新属性
void set(const std::string& key, const std::string& value) {
properties_[key] = value;
}
// 获取属性值
std::string get(const std::string& key) const {
auto it = properties_.find(key);
if (it != properties_.end()) {
return it->second;
}
throw std::runtime_error("Key not found in the property map.");
}
// 删除属性
void remove(const std::string& key) {
properties_.erase(key);
}
// 检查是否存在特定属性
bool has_key(const std::string& key) const {
return properties_.count(key) > 0;
}
private:
// 私有成员变量,存储键值对
std::unordered_map<std::string, std::string> properties_;
};
```
在这个例子中,我们使用了C++标准库中的`std::unordered_map`作为底层数据结构,因为它的查找性能很好。类的公共接口包括添加(set),获取(get),删除(remove)属性以及检查键是否存在(has_key)的方法。
阅读全文
相关推荐
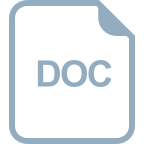
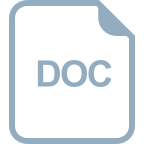
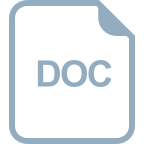
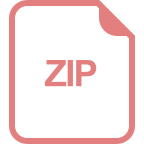
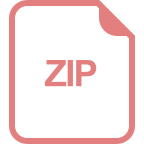
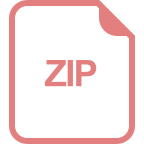
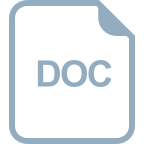
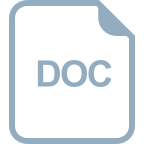
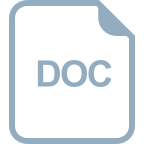
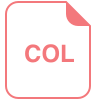
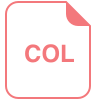






