使用if排列数字大小
时间: 2024-09-26 08:18:51 浏览: 0
在编程中,如果你想使用 if 语句来排列数字大小,通常是在一个数组或集合中。例如,如果你有一个整数数组需要从小到大排序,可以遍历这个数组,通过条件判断进行交换元素的位置。下面是一个简单的示例,用 Java 实现:
```java
int[] numbers = {5, 2, 8, 1, 9}; // 需要排序的数组
// 假设数组长度大于 1
for (int i = 0; i < numbers.length - 1; i++) {
for (int j = 0; j < numbers.length - 1 - i; j++) {
if (numbers[j] > numbers[j + 1]) {
// 交换 numbers[j] 和 numbers[j+1]
int temp = numbers[j];
numbers[j] = numbers[j + 1];
numbers[j + 1] = temp;
}
}
}
System.out.println(Arrays.toString(numbers)); // 输出 [1, 2, 5, 8, 9]
相关问题
使用js代码的if,把三个数字的大小排列一下
我可以回答这个问题。以下是一个使用 JavaScript 的 if 语句来排列三个数字大小的示例代码:
```
let num1 = 5;
let num2 = 2;
let num3 = 8;
if (num1 > num2 && num1 > num3) {
if (num2 > num3) {
console.log(num1, num2, num3);
} else {
console.log(num1, num3, num2);
}
} else if (num2 > num1 && num2 > num3) {
if (num1 > num3) {
console.log(num2, num1, num3);
} else {
console.log(num2, num3, num1);
}
} else if (num3 > num1 && num3 > num2) {
if (num1 > num2) {
console.log(num3, num1, num2);
} else {
console.log(num3, num2, num1);
}
}
```
这段代码将三个数字进行比较,并按照从大到小的顺序输出它们。在这个示例中,数字 5、2 和 8 将被输出为 8、5、2。
用c语言编码一个排列数字大小的程序
你好,我可以回答这个问题。以下是一个用 C 语言编写的排列数字大小的程序示例:
#include <stdio.h>
int main() {
int nums[10] = {5, 2, 9, 1, 3, 6, 8, 7, 4, 0};
int i, j, temp;
for (i = 0; i < 10; i++) {
for (j = i + 1; j < 10; j++) {
if (nums[i] > nums[j]) {
temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
}
printf("排列后的数字为:");
for (i = 0; i < 10; i++) {
printf("%d ", nums[i]);
}
printf("\n");
return 0;
}
这个程序使用了冒泡排序算法,将一个包含 10 个数字的数组按照从小到大的顺序排列,并输出排列后的结果。希望这个程序能够帮到你。
相关推荐
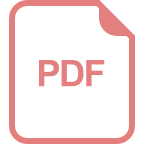
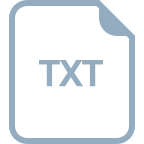
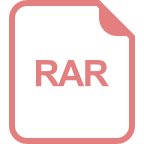












