编写一个程序,用户输入若干学生的学号、成绩,程序建立一个学生链表,并显示该学生表。
时间: 2024-09-14 15:14:38 浏览: 39
编写这样一个程序通常涉及到以下几个步骤:
1. 定义一个学生节点类(Student Node),包含学号(studentID)和成绩(score)两个属性,以及指向下一个节点的指针。
```python
class StudentNode:
def __init__(self, student_id, score):
self.student_id = student_id
self.score = score
self.next = None
```
2. 创建一个空的学生链表头结点(head):
```python
def create_empty_student_list():
return StudentNode(None, None)
```
3. 提供函数让用户输入学生信息并添加到链表中:
```python
def add_student_to_list(list_head, student_id, score):
new_node = StudentNode(student_id, score)
if list_head is None:
list_head = new_node
else:
current = list_head
while current.next is not None:
current = current.next
current.next = new_node
return list_head
```
4. 显示学生链表的内容:
```python
def display_student_list(list_head):
current = list_head
if current is not None:
print(f"学号\t成绩")
while current is not None:
print(f"{current.student_id}\t{current.score}")
current = current.next
else:
print("学生列表为空。")
```
5. 主程序循环接收用户输入并调用上述函数:
```python
if __name__ == "__main__":
student_list = create_empty_student_list()
while True:
student_id = input("请输入学生的学号(输入 'q' 结束):")
if student_id.lower() == 'q':
break
score = float(input("请输入学生的成绩:"))
student_list = add_student_to_list(student_list, student_id, score)
display_student_list(student_list)
```
在这个程序中,用户可以持续输入学生的学号和成绩,直到选择退出。每次输入后,新数据都会被添加到链表中,并在结束后展示整个学生列表。
阅读全文
相关推荐
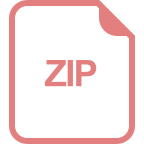
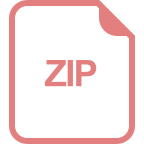
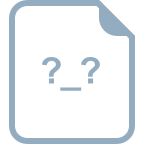
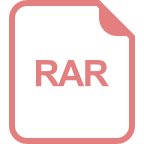
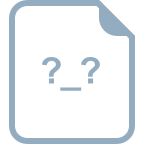
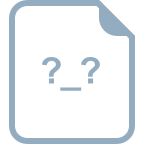
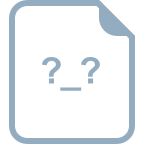
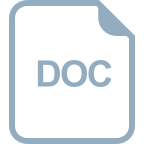
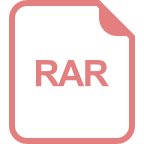
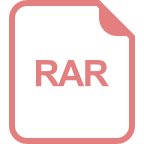
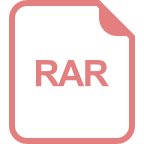
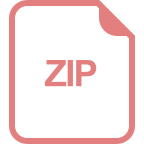
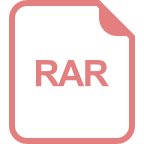
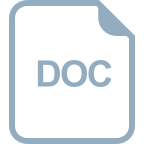





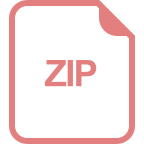