用菜名查找菜品C++用文件形式
时间: 2024-11-15 12:32:37 浏览: 0
在C++中,如果你想要通过菜品名称查找菜品信息并存储在一个文件中,你可以采用以下步骤:
1. **设计数据结构**:
- 创建一个`Menu`类或者简单地使用关联数组(如map),其中键是菜品名称,值是菜品的具体信息(例如字符串、结构体等)。
```cpp
struct MenuItem {
std::string name;
// 其他菜品信息...
};
std::map<std::string, MenuItem> menuItems;
```
2. **读取文件**:
- 编写一个函数来从文件中读取菜品信息,并添加到`menuItems`中。假设文件格式是每行一条记录,菜品名称在第一列。
```cpp
void loadMenuFromFile(const std::string& filename) {
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
size_t pos = line.find(",");
if (pos != std::string::npos) {
std::string name = line.substr(0, pos);
// 提取其他信息,如切割字符串或解析成结构体
MenuItem item = {name, ...};
menuItems[name] = item;
}
}
file.close();
} else {
std::cerr << "Failed to open the file." << std::endl;
}
}
```
3. **查找菜品**:
- 用户可以通过菜品名称查询菜单,使用`menuItems`查找对应的数据。
```cpp
MenuItem findItem(const std::string& itemName) {
auto it = menuItems.find(itemName);
if (it != menuItems.end()) {
return it->second;
}
throw std::runtime_error("Item not found in the menu.");
}
```
4. **输出或处理**:
- 查找菜品后,可以打印信息,或者用于进一步的处理操作。
```cpp
void displayItem(const MenuItem& item) {
std::cout << "Name: " << item.name << ", Info: ... \n";
}
int main() {
loadMenuFromFile("recipes.txt");
MenuItem requestedItem = findItem("宫保鸡丁");
displayItem(requestedItem);
// 等等...
return 0;
}
```
阅读全文
相关推荐
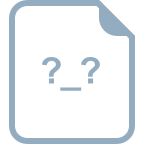
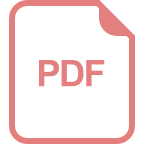
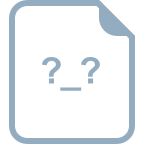













