c++ 命令行参数 -t
时间: 2024-06-10 08:04:13 浏览: 169
在C/C++代码中支持命令行参数,并根据获取到的参数值来进行代码分支的选择可以通过以下几种方法实现:
1. 使用C库的getopt()函数来处理短选项的命令行参数,如-h、-d等。getopt()函数会解析命令行参数并返回相应的选项和参数值。
2. 使用C库的getopt_long()函数来处理长选项和短选项的命令行参数,如--help等。getopt_long()函数可以处理更多的选项,并提供更灵活的选项语法。
3. 根据argc和argv进行命令行参数处理。在程序执行的入口函数int main(int argc, char* argv[])中,argc表示命令行参数的个数,argv是一个指向字符串数组的指针,其中存储了命令行参数的值。
回答完问题后的
相关问题
如何在C++中有效地将命令行参数转换为宽字符数组( CommandLineToArgvW 函数)?
在C++中,你可以使用Windows API函数`CommandLineToArgvW`来有效地将命令行参数转换为宽字符数组。这个函数通常在处理批处理或命令行程序时很有用。下面是基本步骤:
1. **包含头文件**:
首先,你需要包含`shellapi.h`头文件,它包含了`CommandLineToArgvW`函数的声明。
```cpp
#include <windows.h>
```
2. **获取命令行参数**:
使用`GetCommandLineW()`函数获取当前进程的命令行字符串,然后传给`CommandLineToArgvW`。
```cpp
wchar_t* cmdLine = GetCommandLineW();
int argc;
LPWSTR* argv = CommandLineToArgvW(cmdLine, &argc);
```
`argc`变量将存储命令行参数的数量,而`argv`是一个指向宽字符字符串数组的指针,每个元素对应一个命令行参数。
3. **处理数组**:
现在你可以遍历这个数组,对每个参数进行操作。注意,数组的最后一个元素通常是空字符`L'\0'`,表示结束。
```cpp
for (int i = 0; i < argc; ++i) {
wprintf(L"参数 %d: %ls\n", i, argv[i]);
}
```
4. **释放内存**:
当你完成处理后,别忘了使用`LocalFree()`函数释放`argv`所指向的内存。
```cpp
LocalFree(argv);
```
用命令行参数如何实现,举例来说
使用命令行参数实现在DLL中接收来自EXE的数据非常直观。当用户启动EXE时,可以包含一串可自定义的参数,这些参数会被自动传递给执行的程序,包括DLL。下面是一个简单的例子说明这个过程:
假设我们有一个名为`MyExe.exe`的EXE程序,它需要调用名为`MyDll.dll`的DLL,传递两个参数:`message`和`number`。在EXE的main函数里,你可以这样调用:
```cpp
// EXE部分 (C++示例)
#include <windows.h>
#include "MyDll.h" // 如果是静态链接,包含头文件
int main(int argc, char* argv[]) {
if (argc != 3) {
std::cerr << "Usage: MyExe.exe message number\n";
return 1;
}
const wchar_t* message = argv[1]; // 第一个参数
int number = _wtoi(argv[2]); // 将第二个字符串参数转换为整数
// 调用DLL中的某个函数,比如显示消息
DisplayMessage(message, number);
return 0;
}
```
在DLL中,`DisplayMessage`函数可能会长这样:
```cpp
// DLL部分 (同样C++示例)
extern "C" __declspec(dllexport) void DisplayMessage(const wchar_t* message, int number) {
// 在这里处理message和number
wprintf(L"Received message: %ls, number: %d\n", message, number);
}
```
当你运行`MyExe.exe hello 42`时,`MyDll.dll`会接收到`message="hello"`和`number=42`这两个参数。这种方法适合于传递简单数据和少量参数,但对于复杂的结构化数据,可能还需要额外的编码和解码机制。
阅读全文
相关推荐
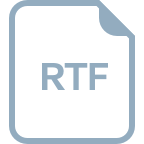















