编写一个程序,可以在命令行输入参数,完成指定文件的缩放,并存储到新文件,命令行参数如下 zoom file1.bmp 200 file2.bmp 第一个参数为可执行程序名称,第二个参数为原始图像文件名,第三个参数为缩放比例(百分比),第四个参数为新文件名。c++
时间: 2024-03-18 18:40:43 浏览: 71
以下是使用 C++ 实现命令行参数缩放图片的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <cmath>
using namespace std;
#pragma pack(push, 1)
// BMP 文件头
struct BMPFileHeader {
uint16_t file_type; // 文件类型,必须为 "BM"
uint32_t file_size; // 文件大小,字节为单位
uint16_t reserved1; // 保留字段,必须为 0
uint16_t reserved2; // 保留字段,必须为 0
uint32_t offset; // 数据偏移量,字节为单位
};
// BMP 信息头
struct BMPInfoHeader {
uint32_t header_size; // 信息头大小
int32_t width; // 图像宽度,像素为单位
int32_t height; // 图像高度,像素为单位
uint16_t planes; // 图像平面数,必须为 1
uint16_t bits_per_pixel; // 每像素位数
uint32_t compression; // 压缩方式
uint32_t image_size; // 图像大小,字节为单位
int32_t x_pixels_per_m; // 水平分辨率,像素为单位
int32_t y_pixels_per_m; // 垂直分辨率,像素为单位
uint32_t colors_used; // 使用的颜色数
uint32_t colors_important; // 重要的颜色数
};
#pragma pack(pop)
// 缩放图片
void zoom_image(char* input_file, int scale, char* output_file) {
// 打开输入文件
ifstream fin(input_file, ios::binary);
if (!fin) {
cerr << "Error: failed to open input file: " << input_file << endl;
return;
}
// 读取 BMP 文件头
BMPFileHeader file_header;
fin.read((char*)&file_header, sizeof(file_header));
if (file_header.file_type != 0x4D42) {
cerr << "Error: invalid BMP file format" << endl;
return;
}
// 读取 BMP 信息头
BMPInfoHeader info_header;
fin.read((char*)&info_header, sizeof(info_header));
if (info_header.bits_per_pixel != 24) {
cerr << "Error: unsupported color depth" << endl;
return;
}
// 计算缩放后的图像大小
int new_width = round(info_header.width * scale / 100.0);
int new_height = round(info_header.height * scale / 100.0);
int new_image_size = new_width * new_height * 3;
// 创建输出文件
ofstream fout(output_file, ios::binary);
if (!fout) {
cerr << "Error: failed to create output file: " << output_file << endl;
return;
}
// 写入 BMP 文件头
BMPFileHeader new_file_header = file_header;
new_file_header.file_size = sizeof(new_file_header) + sizeof(info_header) + new_image_size;
new_file_header.offset = sizeof(new_file_header) + sizeof(info_header);
fout.write((char*)&new_file_header, sizeof(new_file_header));
// 写入 BMP 信息头
BMPInfoHeader new_info_header = info_header;
new_info_header.width = new_width;
new_info_header.height = new_height;
new_info_header.image_size = new_image_size;
fout.write((char*)&new_info_header, sizeof(new_info_header));
// 缩放图像数据
int row_padding = (4 - (info_header.width * 3 % 4)) % 4;
int new_row_padding = (4 - (new_width * 3 % 4)) % 4;
char* row_buffer = new char[info_header.width * 3];
char* new_row_buffer = new char[new_width * 3];
for (int y = 0; y < info_header.height; y++) {
// 读取一行像素数据
fin.read(row_buffer, info_header.width * 3);
// 缩放像素数据并写入输出文件
for (int x = 0; x < info_header.width; x++) {
int new_x = round(x * scale / 100.0);
int new_y = round(y * scale / 100.0);
int index = y * info_header.width * 3 + x * 3;
int new_index = new_y * new_width * 3 + new_x * 3;
new_row_buffer[new_index] = row_buffer[index];
new_row_buffer[new_index + 1] = row_buffer[index + 1];
new_row_buffer[new_index + 2] = row_buffer[index + 2];
}
// 写入一行缩放后的像素数据
fout.write(new_row_buffer, new_width * 3);
fout.write(string(new_row_padding, 0).c_str(), new_row_padding);
}
// 关闭文件和释放内存
fin.close();
fout.close();
delete[] row_buffer;
delete[] new_row_buffer;
}
int main(int argc, char* argv[]) {
// 检查命令行参数
if (argc != 5) {
cerr << "Usage: " << argv[0] << " input_file scale output_file" << endl;
return 1;
}
// 解析命令行参数
char* input_file = argv[1];
int scale = atoi(argv[2]);
char* output_file = argv[3];
// 缩放图片并保存到输出文件
zoom_image(input_file, scale, output_file);
return 0;
}
```
该程序使用了 BMP 文件格式,因此只能缩放 BMP 格式的图片。如果要支持其他格式的图片,需要使用不同的文件格式和读取方式。
阅读全文
相关推荐
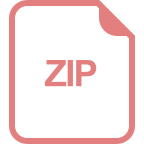
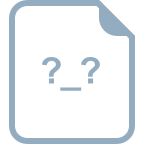
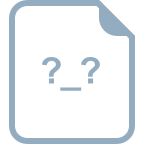









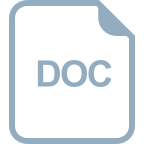
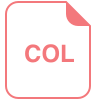

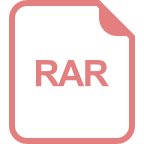
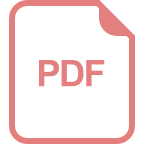
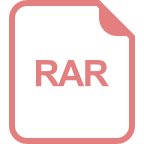