编写一个C语言程序,可以在命令行输入参数,完成指定文件的缩放,并存储到新文件,命令行参数如下: zoom file1.bmp 200 file2.bmp 第一个参数为可执行程序名称,第二个参数为原始图像文件名,第三个参数为缩放比例(百分比),第四个参数为新文件名。
时间: 2024-02-24 10:54:11 浏览: 67
好的,以下是一个简单的C语言程序示例,可以实现您的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include "bmp.h"
int main(int argc, char *argv[]) {
if (argc != 5) {
printf("Usage: zoom file1.bmp 200 file2.bmp\n");
return 1;
}
char *filename_in = argv[1];
float scale = atof(argv[2]) / 100.0;
char *filename_out = argv[4];
BMPImage image;
if (!readBMPImage(&image, filename_in)) {
printf("Unable to open file: %s\n", filename_in);
return 1;
}
BMPImage new_image;
new_image.width = (int) round(image.width * scale);
new_image.height = (int) round(image.height * scale);
new_image.pixels = malloc(new_image.width * new_image.height * sizeof(Pixel));
if (new_image.pixels == NULL) {
printf("Unable to allocate memory.\n");
return 1;
}
for (int i = 0; i < new_image.height; i++) {
for (int j = 0; j < new_image.width; j++) {
int x = (int) round(j / scale);
int y = (int) round(i / scale);
new_image.pixels[i * new_image.width + j] = image.pixels[y * image.width + x];
}
}
if (!writeBMPImage(&new_image, filename_out)) {
printf("Unable to save file: %s\n", filename_out);
return 1;
}
free(image.pixels);
free(new_image.pixels);
printf("Image resized and saved successfully.\n");
return 0;
}
```
该程序使用了 bmp.h 头文件,该文件可以在网上下载。您只需要将代码保存为 zoom.c,使用 gcc 编译即可生成可执行文件。在命令行中输入 "./zoom file1.bmp 200 file2.bmp",即可完成图片缩放并保存到指定文件。
阅读全文
相关推荐
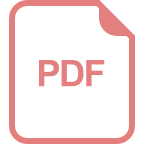
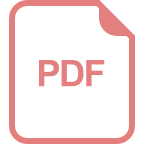






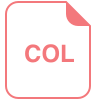
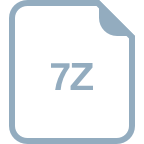
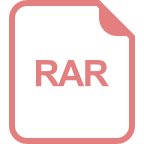
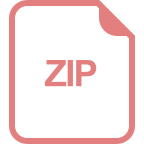
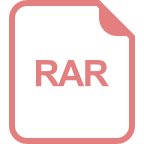
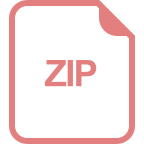
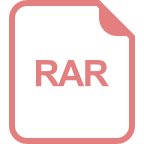
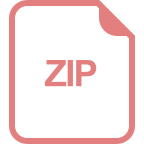
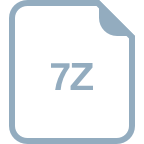
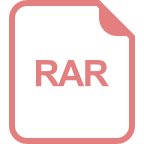