高精度除法C++代码普通方法实现
时间: 2024-10-14 09:08:01 浏览: 37
在C++中,高精度除法通常涉及到大整数运算,因为标准库提供的`std::div`或`std::fdiv`函数并不能处理任意精度的大整数。你可以通过手动实现长除法算法或者使用第三方库如GMP(GNU Multiple Precision Arithmetic Library)来完成这个任务。
下面是一个基于手算长除法思想的简单示例,假设我们有一个自定义的`BigInt`类:
```cpp
class BigInt {
public:
// ... 定义存储大整数的数据结构和基本操作,比如加减乘等
private:
static BigInt divide(BigInt num, BigInt divisor) {
BigInt quotient;
BigInt remainder;
// 初始化结果和余数
quotient = BigInt(0);
remainder = num;
while (remainder.abs() >= divisor.abs()) { // abs()获取绝对值
BigInt shift = divisor; // 防止除数变负
if (shift > remainder) {
shift = shift - remainder;
}
quotient += shift / divisor;
remainder -= shift * divisor; // 更新余数
}
return quotient;
}
};
BigInt bigDivide(const BigInt& num, const BigInt& divisor) {
// 处理正负号
bool isNegative = (num.sign() != divisor.sign());
BigInt result = divide(std::abs(num), std::abs(divisor));
// 根据原数的正负调整结果的正负
if (isNegative && !result.sign()) {
result = -result;
} else if (!isNegative && result.sign()) {
result = -result;
}
return result;
}
```
这个代码定义了一个简单的高精度除法函数`bigDivide`,它首先计算两个数的绝对值并执行长除法,然后根据原始数的正负号调整结果的正负。
阅读全文
相关推荐
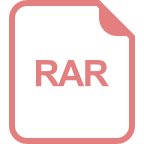
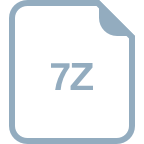
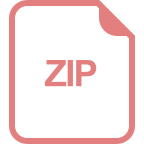












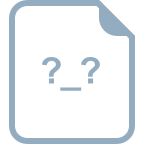
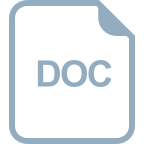
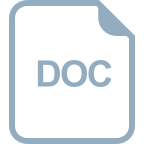
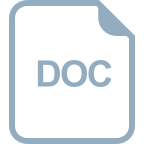