15 个数存放在一个数组中,输入一个数,要求查找该数是数组中第几个元素的值。c语言
时间: 2024-12-08 11:16:51 浏览: 11
在C语言中,你可以使用线性搜索的方式来查找给定数值在数组中的位置。以下是简单的步骤:
1. 定义一个函数,例如`findElementIndex`,接受两个参数:数组`arr`和要查找的数`target`,以及数组的长度`length`。
```c
int findElementIndex(int arr[], int target, int length) {
for (int i = 0; i < length; i++) {
if (arr[i] == target) {
return i;
}
}
// 如果找不到目标值,返回 -1 或者一个表示未找到的错误代码
return -1;
}
```
2. 调用这个函数,传递数组、目标值和数组长度,得到对应的索引。
```c
int main() {
int arr[15] = {...}; // 填充你的数组
int target = ...; // 用户输入或已知的目标数值
int array_length = sizeof(arr) / sizeof(arr[0]);
int index = findElementIndex(arr, target, array_length);
if (index != -1) {
printf("Number %d is at index %d in the array.\n", target, index);
} else {
printf("Number %d not found in the array.\n", target);
}
return 0;
}
```
在这个示例中,函数会遍历整个数组,一旦找到目标值就立即返回其索引。如果遍历结束后仍未找到,返回-1表明目标值不存在于数组中。
阅读全文
相关推荐
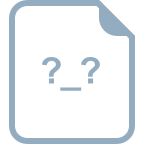
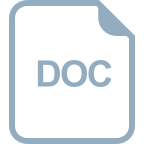
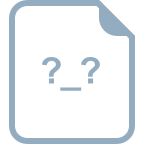















