使用面向对象设计(java语言),实现上下文关键字(KWIC)检索系统: 系统接受多行输入 每行有若干词 每个词由若干字符组成 每行都可以循环移位:重复地把第一个词删除,然后接到行末 KWIC把所有行的各种移位情况按照字母表顺序输出
时间: 2024-10-08 21:12:03 浏览: 92
在Java中,实现一个基于面向对象的设计来构建KWIC(Keyword in Context)检索系统,我们可以创建以下几个关键类:
1. **Word** 类:代表文本中的单个词,包含字符数组、长度属性以及构造函数用于初始化。
```java
public class Word {
private char[] characters;
private int length;
public Word(char[] characters) {
this.characters = characters;
this.length = characters.length;
}
// getter and setter methods
}
```
2. **Line** 类:表示文本行,包含一个Word数组和移位操作的方法。
```java
public class Line {
private Word[] words;
public Line(Word[] words) {
this.words = words;
}
// shift() method to cycle the words in the line
public void shift() {
if (words.length > 0) {
Word first = words[0];
words = Arrays.copyOfRange(words, 1, words.length);
words = new Word[]{first}.concat(words);
}
}
// toString() method for outputting the line
@Override
public String toString() {
return Joiner.on(" ").join(words);
}
}
```
3. **KWICSystem** 类:负责接收输入、处理线性数据并按照字母顺序输出各种移位情况。
```java
import java.util.*;
public class KWICSystem {
public List<String> getKwic(String[][] input) {
ArrayList<String> kwicOutput = new ArrayList<>();
for (String[] inputLine : input) {
Word[] words = convertToWords(inputLine);
Line line = new Line(words);
generateKwic(line, kwicOutput);
}
Collections.sort(kwicOutput); // Sort by word's first character
return kwicOutput;
}
// Convert input string to Word objects
private Word[] convertToWords(String[] inputLine) {
// implementation...
}
// Generate all possible KWIC contexts for a line
private void generateKwic(Line line, List<String> kwicOutput) {
// implementation with loop through shifting and adding to output
}
// ... other helper methods as needed
}
```
在这个结构中,用户可以提供多行文本作为输入给`KWICSystem`实例,它会生成并返回所有可能的KWIC输出,按字母顺序排列。
阅读全文
相关推荐
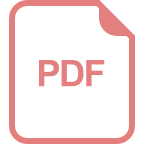
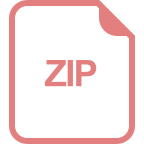
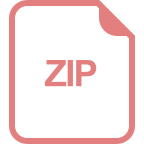











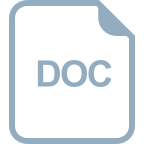
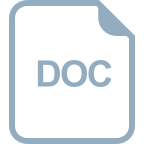
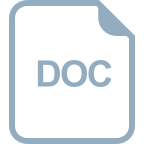
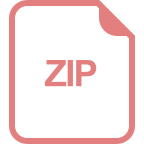
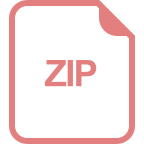
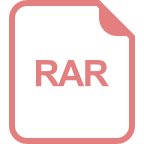