c++20 读写csv文件
时间: 2024-09-04 22:01:07 浏览: 100
C++20并没有直接提供内置的CSV文件处理功能,但你可以使用第三方库如`csv-parser`、`nlohmann::basic_csv`或`fmt::format`等来方便地进行CSV文件的读写操作。以下是一个简单的例子,使用`std::fstream`配合`std::string_view`和`std::getline`来解析CSV,以及`fmt::format`来进行写入:
**读取CSV(示例)**:
```cpp
#include <fstream>
#include <sstream>
#include <vector>
#include <string_view>
#include <fmt/core.h>
std::vector<std::vector<std::string>> read_csv(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file");
}
std::vector<std::vector<std::string>> data;
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
std::vector<std::string> row;
std::string cell;
while (iss >> cell) {
row.push_back(cell);
// 检查是否到了新的一行,如果没有就添加逗号
if (!(iss.peek() == ',' || iss.peek() == '\n')) {
iss.putback(',');
}
}
data.push_back(row);
}
return data;
}
// 使用示例
int main() {
try {
auto csv_data = read_csv("data.csv");
for (const auto& row : csv_data) {
fmt::print("{}\n", row);
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << "\n";
}
return 0;
}
```
**写入CSV(示例)**:
```cpp
void write_csv(const std::vector<std::vector<std::string>>& data, const std::string& filename) {
std::ofstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to create the output file");
}
for (const auto& row : data) {
for (size_t i = 0; i < row.size(); ++i) {
if (i > 0) {
file << ",";
}
file << row[i];
}
file << "\n";
}
}
// 使用示例
int main() {
std::vector<std::vector<std::string>> data = {{"Name", "Age", "City"}, {"Alice", "25", "New York"}, {"Bob", "30", "San Francisco"}};
write_csv(data, "output.csv");
return 0;
}
```
请注意,这些示例非常基础,实际使用时可能需要处理更复杂的边界情况,比如错误处理、文件编码等。
阅读全文
相关推荐
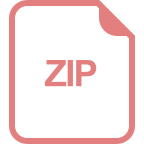
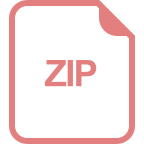
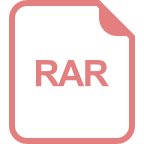
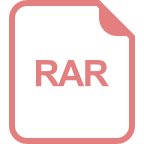





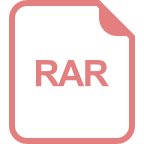
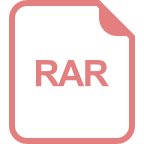
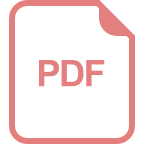
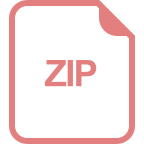



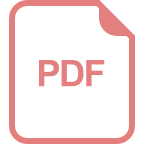
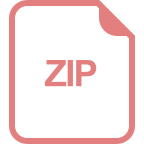