java实现三维散点曲面插值
时间: 2023-11-29 17:05:08 浏览: 73
根据提供的引用内容,我们可以使用Java中的Apache Commons Math库来实现三维散点曲面插值。具体步骤如下:
1. 首先,需要在项目中引入Apache Commons Math库。可以在Maven项目中添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-math3</artifactId>
<version>3.6.1</version>
</dependency>
```
2. 创建一个三维散点数据集。可以使用`RealVector`对象来表示三维坐标点,然后将这些点添加到`ArrayList`中。
```java
import org.apache.commons.math3.analysis.interpolation.TricubicInterpolator;
import org.apache.commons.math3.analysis.interpolation.TricubicSplineInterpolatingFunction;
import org.apache.commons.math3.analysis.interpolation.WeightFunction;
import org.apache.commons.math3.analysis.interpolation.WeightedEvaluation;
import org.apache.commons.math3.analysis.interpolation.WeightedObservedPoint;
import org.apache.commons.math3.analysis.interpolation.WeightedObservedPoints;
import java.util.ArrayList;
import java.util.List;
public class InterpolationExample {
public static void main(String[] args) {
// 创建一个三维散点数据集
List<WeightedObservedPoint> points = new ArrayList<>();
points.add(new WeightedObservedPoint(1, new double[]{0, 0, 0}, 1));
points.add(new WeightedObservedPoint(2, new double[]{0, 0, 1}, 2));
points.add(new WeightedObservedPoint(3, new double[]{0, 1, 0}, 3));
points.add(new WeightedObservedPoint(4, new double[]{0, 1, 1}, 4));
points.add(new WeightedObservedPoint(5, new double[]{1, 0, 0}, 5));
points.add(new WeightedObservedPoint(6, new double[]{1, 0, 1}, 6));
points.add(new WeightedObservedPoint(7, new double[]{1, 1, 0}, 7));
points.add(new WeightedObservedPoint(8, new double[]{1, 1, 1}, 8));
// 创建三次立方插值器
TricubicInterpolator interpolator = new TricubicInterpolator();
// 使用三次立方插值器拟合数据
TricubicSplineInterpolatingFunction function = interpolator.interpolate(points);
// 计算插值点的值
double[] point = new double[]{0.5, 0.5, 0.5};
double value = function.value(point);
System.out.println("插值点的值为:" + value);
}
}
```
在上面的代码中,我们创建了一个包含8个三维坐标点的数据集,并使用`TricubicInterpolator`类创建了一个三次立方插值器。然后,我们使用插值器拟合数据,并计算了一个插值点的值。
3. 可以使用`TricubicInterpolator`类的`interpolate`方法来设置插值器的参数。例如,可以使用`WeightFunction`类来设置权重函数,以便更好地拟合数据。
```java
// 创建三次立方插值器
TricubicInterpolator interpolator = new TricubicInterpolator();
// 设置权重函数
WeightFunction weightFunction = new WeightFunction() {
@Override
public double value(double[] point) {
double x = point[0];
double y = point[1];
double z = point[2];
return 1 / (1 + x * x + y * y + z * z);
}
};
interpolator.setWeightFunction(weightFunction);
// 使用三次立方插值器拟合数据
TricubicSplineInterpolatingFunction function = interpolator.interpolate(points);
```
在上面的代码中,我们使用`WeightFunction`类创建了一个权重函数,并将其设置为插值器的参数。这个权重函数将会使插值器更好地拟合数据。
相关推荐
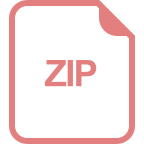
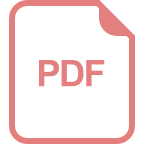
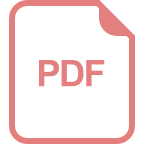














