module 'torch.nn' has no attribute 'linear'
时间: 2023-10-26 09:07:01 浏览: 218
这个错误提示表明在 torch.nn 模块中没有名为 linear 的属性。通常这是因为没有正确导入所需的模块或类。在使用 linear 层之前,需要先导入 torch.nn 模块并创建一个 nn.Module 的子类,然后在该子类中定义 linear 层。
以下是一个示例代码:
```
import torch
import torch.nn as nn
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.linear = nn.Linear(10, 1)
def forward(self, x):
out = self.linear(x)
return out
```
在这个示例中,我们首先导入了 torch 和 torch.nn 模块。然后,我们创建了一个名为 MyModel 的 nn.Module 子类,并在其中定义了一个名为 linear 的线性层。最后,我们实现了 forward 方法,该方法接受输入张量 x 并返回输出张量 out。
相关问题
AttributeError: mAttributeError: module 'torch.nn' has no attribute 'SiLU'odule torch.nn has no attribute SiLU
`AttributeError: module 'torch.nn' has no attribute 'SiLU'` 这是一个常见的Python错误,通常发生在尝试使用PyTorch库中的功能时。这个错误意味着你在尝试访问`torch.nn`模块中不存在的'SiLU'属性或函数。`SiLU`,也称为Sigmoid Linear Unit,是一种激活函数,在某些版本的PyTorch更新之前可能是缺失的,或者你需要先导入正确的子模块。
要解决这个问题,你可以按照以下步骤检查:
1. 确认是否已经安装了包含SiLU函数的最新版本的PyTorch。如果是早期版本,可以尝试升级到支持SiLU的版本。
```bash
pip install torch torchvision -U
```
2. 检查你的代码中是否正确地引入了`nn.SiLU()`。确保在使用它之前导入了`torch.nn.functional`,因为`SiLU`通常在这个模块里。
```python
import torch.nn as nn
from torch.nn import functional as F
# 然后就可以使用 F.silu() 或者 nn.SiLU()
x = F.silu(y)
```
如果以上步骤都确认无误,但仍然报错,那可能是在其他环境中某个地方创建了一个局部的`SiLU`引用,导致全局找不到这个名称。确保在整个项目范围内使用的是同一个`torch.nn`模块。
AttributeError: module 'torch.nn' has no attribute 'linear'
在解决"AttributeError: module 'torch.nn' has no attribute 'linear'"的问题时,您可以尝试以下方法:
1. 检查torch版本:请确保您使用的是最新版本的torch。可以使用以下命令升级torch:
```python
pip install torch --upgrade
```
2. 检查导入的模块:请确保您正确导入了所需的模块。在您的代码中,应该使用`import torch.nn as nn`来导入torch的nn模块。
3. 检查属性名称:请确认您使用正确的属性名称。在您的代码中,应该使用`nn.Linear`而不是`nn.linear`。将您的代码修改为以下形式:
```python
linear_layer = nn.Linear(in_features, out_features)
```
其中,`in_features`和`out_features`应该分别代表输入和输出的特征数量。
4. 检查函数调用:请确保您正确调用了nn.Linear函数。例如,在您的代码中,您可以使用以下方式创建一个线性模型:
```python
linear_model = nn.Linear(ndim, 1)
```
阅读全文
相关推荐
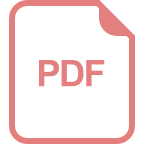
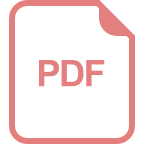
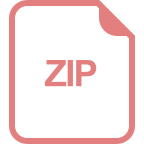













