用数据结构的方法写个代码1.尝试给如下节点构建一颗哈夫曼树,数据为:{H,E,L,O,W,R,D}, 对应的权重为:{1,1,3,2,1,1,1} 2.求取所有字母的哈夫曼编码。
时间: 2024-12-12 18:24:06 浏览: 4
为了构建哈夫曼树并获取每个字符的哈夫曼编码,我们可以采用贪心算法。首先,创建一个优先队列(通常使用最小堆),然后按照权重将字符及其权重插入队列。接下来,我们不断从队列中取出两个权值最小的节点合并成一个新的节点,新节点的权值为其父节点的权值之和,并将这个新节点放回队列中。当只剩下一个节点时,这便是哈夫曼树的根节点。
以下是使用Python编写的一个简单示例:
```python
import heapq
# 数据和权重
data = ['H', 'E', 'L', 'O', 'W', 'R', 'D']
weights = [1, 1, 3, 2, 1, 1, 1]
# 创建堆
heap = [(weight, (char, weight)) for char, weight in zip(data, weights)]
heapq.heapify(heap)
# 合并节点直到只剩一个
while len(heap) > 1:
# 取出两个最小的节点
left_weight, (left_char, left_weight) = heapq.heappop(heap)
right_weight, (right_char, right_weight) = heapq.heappop(heap)
# 创建新的节点并添加回堆
new_node_weight = left_weight + right_weight
new_node = (new_node_weight, (None, (left_char, right_char), new_node_weight))
heapq.heappush(heap, new_node)
# 根节点就是哈夫曼树的构造结果
huffman_tree_root, codes = heap[0][1]
codes_dict = {char: codes[1] if codes[0] is None else codes[0] + codes[1] for char, codes in huffman_tree_root[1].items()}
# 输出所有字母的哈夫曼编码
for char, code in codes_dict.items():
print(f"{char}: {code}")
#
阅读全文
相关推荐
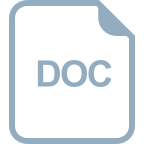
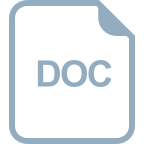
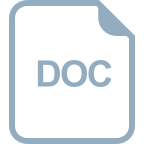




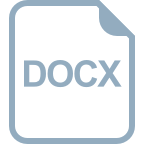
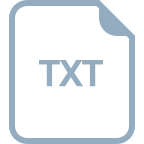
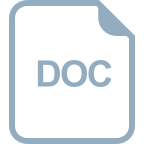
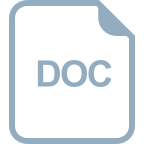
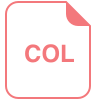
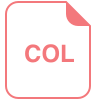
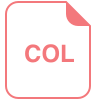
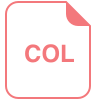
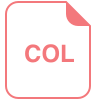



