python 方程求解
时间: 2023-11-22 19:50:56 浏览: 31
以下是三种Python解方程的方法:
1.使用Numpy求解线性方程组
```python
import numpy as np
# 定义系数矩阵A和常数矩阵B
A = np.array([[1, 2], [3, 4]])
B = np.array([5, 6])
# 求解线性方程组
X = np.linalg.solve(A, B)
# 输出解
print(X) # 输出:[-4. 4.5]
```
2.使用SymPy解方程
```python
from sympy import symbols, solve
# 定义未知数
x, y = symbols('x y')
# 定义方程
eq1 = x + 2*y - 5
eq2 = 3*x + 4*y - 6
# 解方程
result = solve((eq1, eq2), (x, y))
# 输出解
print(result) # 输出:{x: -4, y: 4.5}
```
3.使用SciPy解非线性方程组
```python
from scipy.optimize import fsolve
# 定义方程组
def equations(p):
x, y = p
eq1 = x**2 + y**2 - 1
eq2 = x - y**3
return (eq1, eq2)
# 求解方程组
result = fsolve(equations, (1, 1))
# 输出解
print(result) # 输出:[0.72449139 0.6891538 ]
```
相关问题
python方程求解
您好!对于 Python 中的方程求解,有多种方法和库可供选择。以下是一些常见的方法:
1. 符号计算库 SymPy:SymPy 是一个强大的符号计算库,可以用于解析和数值求解方程。您可以使用 SymPy 提供的 solve() 函数来求解方程。示例代码如下:
```python
from sympy import symbols, Eq, solve
# 创建未知数
x = symbols('x')
# 创建方程
equation = Eq(x**2 + 2*x - 8, 0)
# 求解方程
solutions = solve(equation, x)
print(solutions)
```
2. 数值计算库 NumPy:NumPy 是一个强大的数值计算库,可以用于求解数值方程。您可以使用 NumPy 提供的 roots() 函数来求解多项式方程。示例代码如下:
```python
import numpy as np
# 定义方程的系数
coefficients = [1, 2, -8]
# 求解方程
solutions = np.roots(coefficients)
print(solutions)
```
3. 科学计算库 SciPy:SciPy 是一个用于科学计算的库,其中包含了许多数值求解方程的函数。您可以使用 SciPy 提供的 optimize 模块中的 fsolve() 函数来求解非线性方程。示例代码如下:
```python
from scipy.optimize import fsolve
# 定义方程
def equation(x):
return x**2 + 2*x - 8
# 求解方程
solutions = fsolve(equation, 0)
print(solutions)
```
这些是 Python 中常用的方程求解方法和库。您可以根据具体的需求选择合适的方法来解决问题。希望对您有帮助!如果还有其他问题,请随时提问。
python方程求根的二分法
以下是使用二分法求解方程的Python代码示例:
```python
def func(x):
return x**3 - x**2 + x - 1
a = -1
b = 2
fa = func(a)
fb = func(b)
while a <= b:
x0 = (a + b) / 2
fx0 = func(x0)
if abs(fx0) < 10e-6:
print('x0:', x0, fx0, '<10e-6')
print(x0, '是用二分法求解方程的根')
break
if fa * fx0 < 0:
b = x0
fb = fx0
print('解在左侧,a:', a, ' b:', b, ' x0:', x0)
elif fb * fx0 < 0:
a = x0
fa = fx0
print('解在右侧,a:', a, ' b:', b, ' x0:', x0)
```
这段代码中,`func(x)`是要求解的方程,`a`和`b`是方程的两个根,`fa`和`fb`是分别在`a`和`b`处的函数值。在`while`循环中,我们不断将区间一分为二,直到找到一个根的近似值,使得函数值的绝对值小于$10^{-6}$。如果找到了这样的近似值,我们就输出这个近似值,并结束程序。如果没有找到,我们就根据函数值的正负性来缩小区间,直到找到一个根的近似值为止。
相关推荐

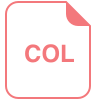
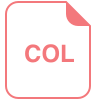

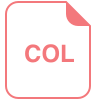







