数据结构进制转换栈c
时间: 2023-11-26 15:45:19 浏览: 89
进制转换栈是一种基于栈的数据结构,用于将一个数从一种进制转换为另一种进制。在进制转换中,我们需要将一个数不断地除以目标进制,将余数依次压入栈中,最后将栈中的元素依次弹出,得到转换后的数。
以下是一个简单的进制转换栈的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == STACK_SIZE - 1;
}
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack overflow\n");
exit(1);
}
s->data[++s->top] = x;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top--];
}
int main() {
Stack s;
int n, base;
printf("Enter a number and its base: ");
scanf("%d %d", &n, &base);
init(&s);
while (n > 0) {
push(&s, n % base);
n /= base;
}
printf("Converted number: ");
while (!is_empty(&s)) {
printf("%d", pop(&s));
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
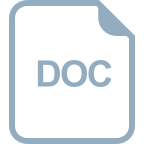
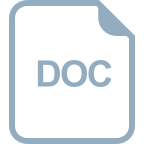
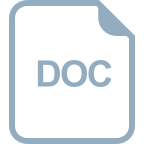
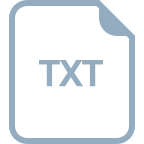

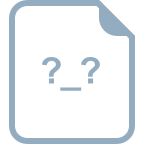
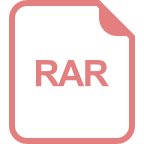
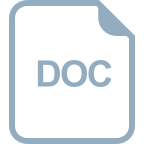








