使用C++语言实现简化RSA,分规则如下: ①实现简化RSA的加解密。 ②需要完整代码 ③使用第三方库:openssl。
时间: 2024-10-16 21:07:08 浏览: 34
在C++中利用OpenSSL库实现简化版的RSA加密解密算法,首先你需要包含必要的头文件并初始化OpenSSL。以下是一个基础示例,展示如何生成公钥、私钥,以及对明文进行加密和解密:
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
// RSA密钥长度 (1024位足够安全)
const int KEY_SIZE = 1024;
class SimplifiedRSA {
public:
// 初始化RSA上下文
SimplifiedRSA() {
rsa = RSA_new();
if (!rsa) {
perror("Failed to create RSA context");
exit(1);
}
RSA_generate_key_ex(rsa, KEY_SIZE, e_gcd, nullptr); // e_gcd是一个用于选择大素数的函数
}
~SimplifiedRSA() {
RSA_free(rsa);
}
// 加密
std::string encrypt(const std::string& plaintext) {
unsigned char ciphertext[rsa->nbits / 8 + 1];
int len = RSA_public_encrypt(strlen(plaintext.c_str()), reinterpret_cast<const unsigned char*>(plaintext.c_str()), ciphertext, rsa, RSA_PKCS1_OAEP_PADDING);
if (len <= 0) {
perror("Encryption failed");
return "";
}
return std::string(reinterpret_cast<char*>(ciphertext), len);
}
// 解密
std::string decrypt(const std::string& ciphertext) {
unsigned char decrypted_data[rsa->nbits / 8 + 1];
int len = RSA_private_decrypt(strlen(ciphertext.c_str()), reinterpret_cast<const unsigned char*>(ciphertext.c_str()), decrypted_data, rsa, RSA_PKCS1_OAEP_PADDING);
if (len <= 0) {
perror("Decryption failed");
return "";
}
return std::string(reinterpret_cast<char*>(decrypted_data), len);
}
private:
RSA* rsa;
};
int main() {
SimplifiedRSA rsa;
std::string plaintext = "Hello, world!";
std::string encrypted_text = rsa.encrypt(plaintext);
std::string decrypted_text = rsa.decrypt(encrypted_text);
if (plaintext == decrypted_text) {
std::cout << "Encryption and decryption successful\n";
} else {
std::cerr << "Decryption error: Original text doesn't match the decrypted text.\n";
}
return 0;
}
```
请注意,此示例仅提供基本功能,并未处理错误处理、输入验证等复杂情况。在实际应用中,你还需要确保妥善保存和管理私钥的安全。
阅读全文
相关推荐
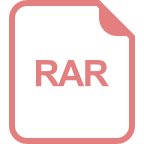
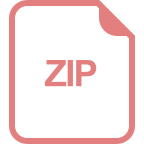
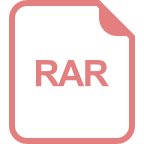

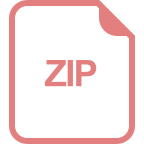
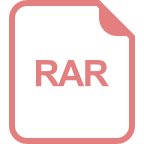
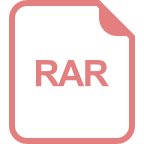
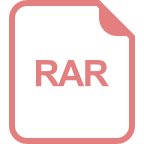
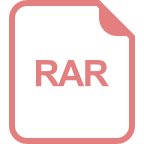
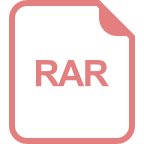
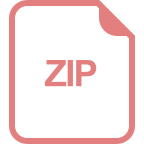
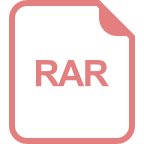
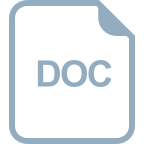
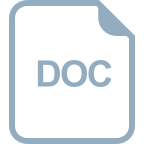
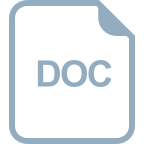
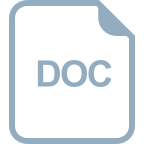
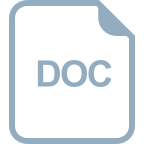
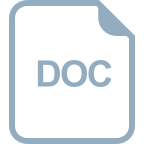
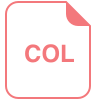
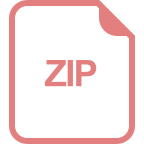