重载3个不同版本的max方法和min方法,这些方法均有3个参数,分别用来求3个int,3个long,3个float数的最大值和最小值,编写java程序,使用这些方法
时间: 2024-10-22 20:21:23 浏览: 35
在Java中,你可以通过方法重载(Method Overloading)的方式,创建三个不同的`max`方法和`min`方法,每个方法接收不同类型的数据作为参数。以下是简单的示例:
```java
public class MathUtils {
// int版 max 方法
public static int max(int a, int b, int c) {
return Math.max(a, Math.max(b, c));
}
// long版 max 方法
public static long max(long a, long b, long c) {
return Math.max(a, Math.max(b, c));
}
// float版 max 方法
public static float max(float a, float b, float c) {
return Math.max(a, Math.max(b, c));
}
// int版 min 方法
public static int min(int a, int b, int c) {
return Math.min(a, Math.min(b, c));
}
// long版 min 方法
public static long min(long a, long b, long c) {
return Math.min(a, Math.min(b, c));
}
// float版 min 方法
public static float min(float a, float b, float c) {
return Math.min(a, Math.min(b, c));
}
}
// 使用例子
public class Main {
public static void main(String[] args) {
int intMax = MathUtils.max(10, 20, 30);
long longMax = MathUtils.max(40L, 50L, 60L);
float floatMax = MathUtils.max(70.0f, 80.0f, 90.0f);
System.out.println("Max of int: " + intMax);
System.out.println("Max of long: " + longMax);
System.out.println("Max of float: " + floatMax);
int intMin = MathUtils.min(1, 2, 3);
long longMin = MathUtils.min(4L, 5L, 6L);
float floatMin = MathUtils.min(7.0f, 8.0f, 9.0f);
System.out.println("Min of int: " + intMin);
System.out.println("Min of long: " + longMin);
System.out.println("Min of float: " + floatMin);
}
}
```
在这个例子中,我们为每种数据类型都定义了一个对应的`max`和`min`方法,可以根据传入的具体数值类型自动选择合适的方法执行。
阅读全文
相关推荐
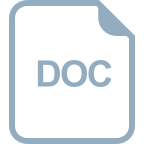
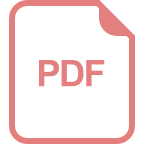




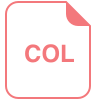

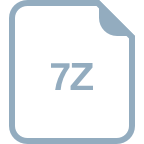
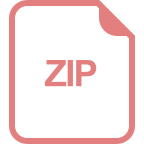
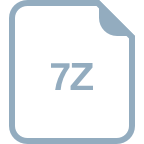
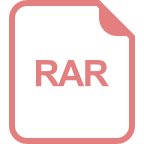
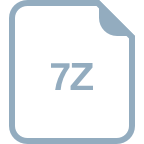
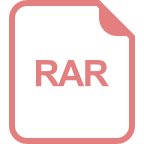
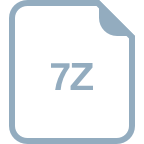