1)重载 3 个不同版本的 max 方法和 min 方法,这些方法均有 3 个参数,分 别用来求 3 个 int、3 个 long、3 个 float 数的最大值和最小值。编写 Java 应用程序, 使用这些方法
时间: 2024-10-23 22:16:53 浏览: 14
在Java中,你可以通过方法重载(Overloading)来创建多个同名但参数列表不同的 `max` 和 `min` 方法,以处理不同类型的数(int、long和float)。以下是简单的实现:
```java
public class NumberComparator {
// int版本的max方法
public static int max(int a, int b, int c) {
return Math.max(a, Math.max(b, c));
}
// long版本的max方法
public static long max(long a, long b, long c) {
return Math.max(a, Math.max(b, c));
}
// float版本的max方法
public static float max(float a, float b, float c) {
return Math.max(a, Math.max(b, c));
}
// 同样的,分别为min方法提供重载版本
// int版本的min方法
public static int min(int a, int b, int c) {
return Math.min(a, Math.min(b, c));
}
// long版本的min方法
public static long min(long a, long b, long c) {
return Math.min(a, Math.min(b, c));
}
// float版本的min方法
public static float min(float a, float b, float c) {
return Math.min(a, Math.min(b, c));
}
public static void main(String[] args) {
System.out.println("Max of integers: " + max(5, 7, 3));
System.out.println("Max of longs: " + max(10L, 20L, 15L));
System.out.println("Max of floats: " + max(1.5f, 2.5f, 1.0f));
System.out.println("Min of integers: " + min(5, 7, 3));
System.out.println("Min of longs: " + min(10L, 20L, 15L));
System.out.println("Min of floats: " + min(1.5f, 2.5f, 1.0f));
}
}
```
在这个例子中,当你在main方法中调用`max`或`min`方法时,编译器会根据传入的实际参数类型自动选择相应的重载版本。
阅读全文
相关推荐
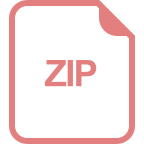
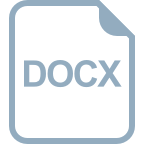
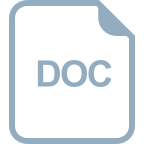















