数据结构中,输入一个数字N,输入n个数创建一个顺序表,然后找出最小值并删除,输出删除后的表源代码C++
时间: 2024-09-24 11:20:42 浏览: 37
在C++中,我们可以使用数组作为顺序表的数据结构,并通过迭代找到最小值并删除。下面是一个简单的示例代码,展示了如何实现这个功能:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点结构体
struct ListNode {
int value;
ListNode* next;
};
// 创建顺序表函数
ListNode* createList(int N, int arr[]) {
ListNode* head = new ListNode{arr[0], nullptr};
ListNode* current = head;
for (int i = 1; i < N; i++) {
current->next = new ListNode{arr[i], nullptr};
current = current->next;
}
return head;
}
// 找到并删除最小值函数
ListNode* deleteMin(ListNode* head) {
if (head == nullptr || head->next == nullptr) {
return head;
}
ListNode* minNode = head;
ListNode* current = head->next;
while (current != nullptr && current->value < minNode->value) {
minNode = current;
current = current->next;
}
// 如果找到了最小值,删除它
if (minNode != head) {
minNode->value = current->value;
ListNode* temp = current->next;
delete current;
minNode->next = temp;
} else {
// 如果头节点是最小值,则直接更新头指针
head = head->next;
}
return head;
}
// 遍历并打印顺序表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != nullptr) {
cout << temp->value << " ";
temp = temp->next;
}
cout << endl;
}
int main() {
int N, num;
cout << "Enter the number of elements: ";
cin >> N;
int arr[N];
cout << "Enter the elements: ";
for (int i = 0; i < N; i++) {
cin >> arr[i];
}
ListNode* list = createList(N, arr);
cout << "Original list before deletion: ";
printList(list);
list = deleteMin(list);
cout << "List after deleting minimum: ";
printList(list);
return 0;
}
```
这段代码首先创建了一个顺序表,然后找到并删除了最小值。最后,它会打印出删除前后的顺序表。
阅读全文
相关推荐
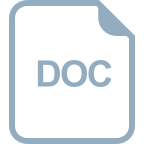
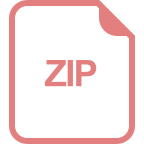
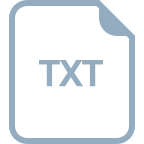

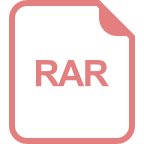
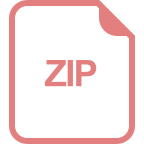
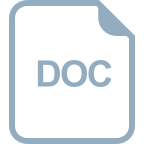
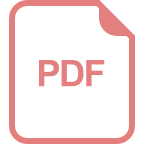
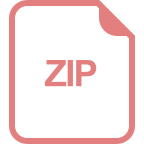
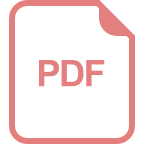
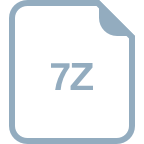
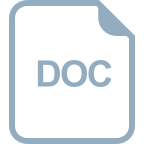
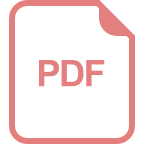
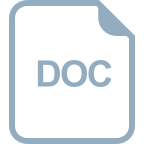
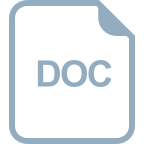
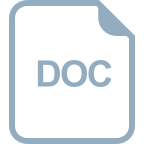
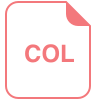
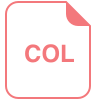
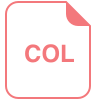