哈夫曼编码压缩图片Python
时间: 2024-05-25 18:08:16 浏览: 102
哈夫曼编码是一种基于最优二叉树的编码方法,可以用于无损压缩数据。在图片压缩方面,哈夫曼编码可以用于对图片像素值进行编码,以实现对图片的压缩。
在Python中,你可以使用Pillow库来读取和处理图片,使用heapq模块来实现哈夫曼树的构建。以下是一个简单的示例代码:
```
from PIL import Image
import heapq
class HuffmanCoding:
def __init__(self, image_path):
self.image_path = image_path
self.image = Image.open(self.image_path)
def get_pixel_frequencies(self):
pixel_frequencies = {}
for pixel in self.image.getdata():
if pixel in pixel_frequencies:
pixel_frequencies[pixel] += 1
else:
pixel_frequencies[pixel] = 1
return pixel_frequencies
def build_huffman_tree(self, pixel_frequencies):
heap = [[weight, [pixel, ""]] for pixel, weight in pixel_frequencies.items()]
heapq.heapify(heap)
while len(heap) > 1:
low = heapq.heappop(heap)
high = heapq.heappop(heap)
for pair in low[1:]:
pair = '0' + pair
for pair in high[1:]:
pair = '1' + pair
heapq.heappush(heap, [low + high] + low[1:] + high[1:])
return sorted(heapq.heappop(heap)[1:], key=lambda p: (len(p[-1]), p))
def get_encoded_image(self, huffman_codes):
encoded_image = ""
for pixel in self.image.getdata():
encoded_image += huffman_codes[pixel]
return encoded_image
def compress_image(self):
pixel_frequencies = self.get_pixel_frequencies()
huffman_codes = dict(self.build_huffman_tree(pixel_frequencies))
encoded_image = self.get_encoded_image(huffman_codes)
return encoded_image
image_path = "test.jpg"
huffman_coding = HuffmanCoding(image_path)
compressed_image = huffman_coding.compress_image()
```
以上代码使用Pillow库打开指定路径的图片,使用get_pixel_frequencies()函数获取每个像素值出现的频率,使用build_huffman_tree()函数构建哈夫曼树并返回编码表,使用get_encoded_image()函数将图片像素值转换为哈夫曼编码,最后使用compress_image()函数返回压缩后的二进制字符串。
阅读全文
相关推荐
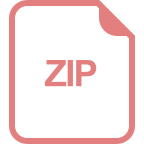
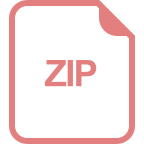


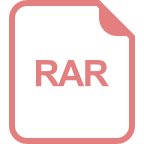
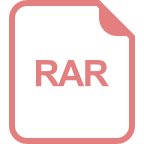
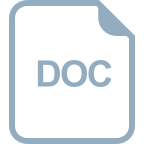
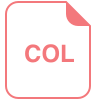
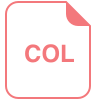
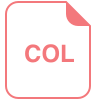
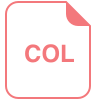
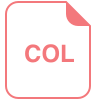
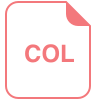
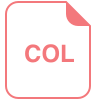
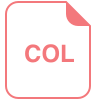
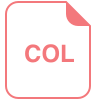