使用面向对象编程思想,模拟超市购物车(利用集合存储),要求实现一下功能: 往购物车中添加商品 在购物车中删除某个商品; 修改购物车中某个商品的数量; 清空购物车; 计算购物车商品的总金额; 将购物车中商品信息存储到本地文件并以购物日期命名文件名
时间: 2024-12-27 12:32:49 浏览: 3
使用面向对象编程的思想,我们可以创建一个名为`ShoppingCart`的类来模拟超市购物车。这里是一个基础的实现:
```java
import java.util.*;
class ShoppingCart {
private Map<String, Integer> items; // 商品ID和数量
public ShoppingCart() {
this.items = new HashMap<>();
}
// 向购物车添加商品
public void addProduct(String productId, int quantity) {
if (!items.containsKey(productId)) {
items.put(productId, quantity);
} else {
items.put(productId, items.get(productId) + quantity);
}
}
// 删除购物车中的商品
public void removeProduct(String productId) {
items.remove(productId);
}
// 修改购物车中商品的数量
public void updateQuantity(String productId, int newQuantity) {
if (items.containsKey(productId)) {
items.put(productId, newQuantity);
}
}
// 清空购物车
public void clearCart() {
items.clear();
}
// 计算购物车商品的总金额
public double calculateTotalPrice(List<CartItem> cartItems) {
return cartItems.stream()
.mapToDouble(cart -> cart.getPrice() * cart.getQuantity())
.sum();
}
// 将购物车信息存储到本地文件
public void saveCartToDisk(Date date) {
try {
File cartFile = new File(date.toString() + ".txt");
BufferedWriter writer = new BufferedWriter(new FileWriter(cartFile));
for (Map.Entry<String, Integer> entry : items.entrySet()) {
writer.write(entry.getKey() + "," + entry.getValue());
writer.newLine();
}
writer.close();
} catch (IOException e) {
System.out.println("Error saving to file: " + e.getMessage());
}
}
// 示例商品信息(用于演示)
static class CartItem {
private String productId;
private int quantity;
private double price;
// 构造函数,getter和setter...
}
}
// 使用示例
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
CartItem apple = new CartItem("apple", 2, 5.99);
cart.addProduct(apple productId, apple.quantity);
// 更多操作...
Date currentDate = new Date();
cart.saveCartToDisk(currentDate);
}
```
阅读全文
相关推荐
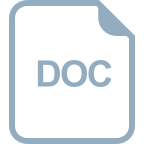
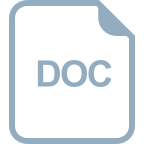
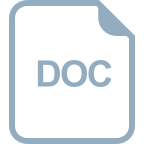




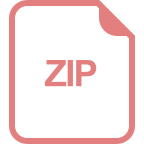
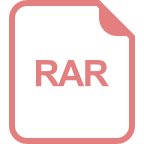
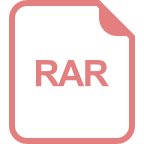
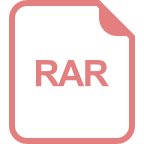
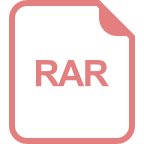
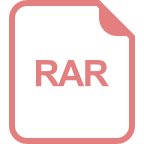
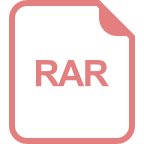
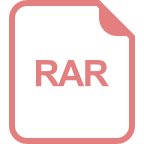
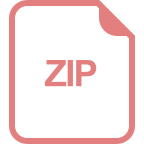
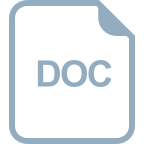
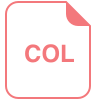
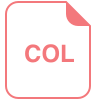