多传感器融合 python
时间: 2023-11-04 13:00:39 浏览: 164
多传感器融合是指将来自不同传感器的数据进行融合和集成,以提高数据的准确性和可靠性。在Python中,可以使用各种库和技术来实现多传感器融合,例如NumPy、SciPy、Pandas和scikit-learn等。以下是一个使用Kalman滤波器进行多传感器融合的示例代码:
```python
import numpy as np
from filterpy.kalman import KalmanFilter
# 初始化Kalman滤波器
kf = KalmanFilter(dim_x=4, dim_z=2)
# 设置状态转移矩阵
kf.F = np.array([[1, 0, 1, 0],
[0, 1, 0, 1],
[0, 0, 1, 0],
[0, 0, 0, 1]])
# 设置测量矩阵
kf.H = np.array([[1, 0, 0, 0],
[0, 1, 0, 0]])
# 设置过程噪声协方差矩阵
kf.Q = np.array([[0.1, 0, 0, 0],
[0, 0.1, 0, 0],
[0, 0, 0.01, 0],
[0, 0, 0, 0.01]])
# 设置测量噪声协方差矩阵
kf.R = np.array([[0.5, 0],
[0, 0.5]])
# 初始化状态和协方差
kf.x = np.array([[0, 0, 0, 0]]).T
kf.P = np.eye(4)
# 传感器数据融合
def sensor_fusion(sensor1_measurement, sensor2_measurement):
# 预测
kf.predict()
# 更新
kf.update(np.array([[sensor1_measurement[0]], [sensor2_measurement[0]]]))
# 获取融合后的状态
fused_state = kf.x[:2]
return fused_state
# 示例传感器数据
sensor1_measurement = [2, 4]
sensor2_measurement = [3, 5]
# 执行传感器数据融合
fused_state = sensor_fusion(sensor1_measurement, sensor2_measurement)
print("融合后的状态:", fused_state)
```
阅读全文
相关推荐
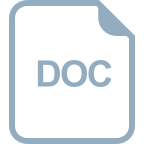
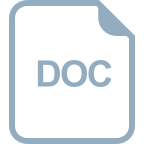
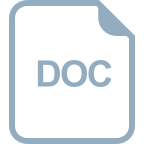
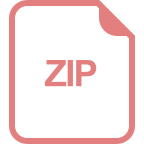
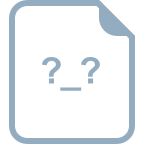
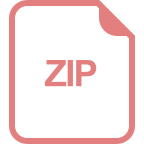
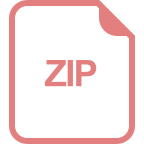
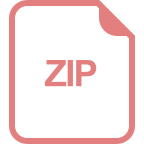
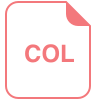
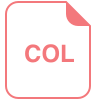
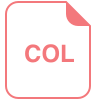
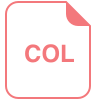
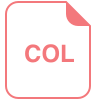
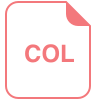
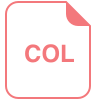
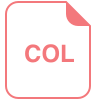
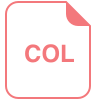

