angular中如何实现监听变量数据的变化
时间: 2024-04-13 17:28:11 浏览: 332
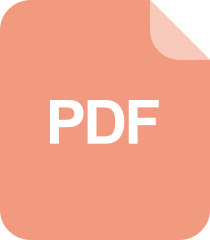
AngularJS监听路由变化的方法
在 Angular 中,你可以使用 `ngOnChanges` 生命周期钩子或 `Observable` 来监听变量数据的变化。
1. 使用 `ngOnChanges` 生命周期钩子:
- 在组件类中实现 `ngOnChanges` 方法,并接收一个 `SimpleChanges` 对象作为参数。
- 在 `ngOnChanges` 方法中,你可以通过访问 `SimpleChanges` 对象获取到变化前后的值,并执行相应的逻辑。
下面是一个示例:
```typescript
import { Component, Input, OnChanges, SimpleChanges } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `
<p>Previous Value: {{ previousValue }}</p>
<p>Current Value: {{ currentValue }}</p>
`
})
export class MyComponent implements OnChanges {
@Input() myVariable: any;
previousValue: any;
currentValue: any;
ngOnChanges(changes: SimpleChanges) {
if (changes.myVariable) {
this.previousValue = changes.myVariable.previousValue;
this.currentValue = changes.myVariable.currentValue;
// 执行其他逻辑...
}
}
}
```
在上面的示例中,`myVariable` 是一个输入变量,当它的值发生变化时,`ngOnChanges` 方法会被调用,并且我们可以通过 `changes.myVariable` 获取到变化前后的值。
2. 使用 `Observable`:
- 在组件中定义一个 `Subject` 或 `BehaviorSubject` 对象来作为可观察对象。
- 在需要监听数据变化的地方,订阅这个可观察对象,并在回调函数中执行相应的逻辑。
下面是一个示例:
```typescript
import { Component } from '@angular/core';
import { Subject } from 'rxjs';
@Component({
selector: 'app-my-component',
template: `
<p>Current Value: {{ currentValue }}</p>
`
})
export class MyComponent {
myVariable$ = new Subject<any>();
currentValue: any;
constructor() {
this.myVariable$.subscribe(value => {
this.currentValue = value;
// 执行其他逻辑...
});
}
updateValue(newValue: any) {
this.myVariable$.next(newValue);
}
}
```
在上面的示例中,`myVariable$` 是一个 `Subject` 对象,我们可以在其他地方调用 `updateValue` 方法来更新变量的值。当值发生变化时,订阅 `myVariable$` 的回调函数会被触发,我们可以在其中获取到最新的值并执行相应的逻辑。
这两种方法各有不同的应用场景,你可以根据实际需求选择合适的方式来监听变量数据的变化。
阅读全文
相关推荐
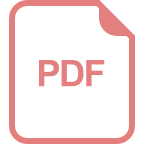
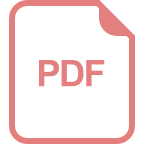
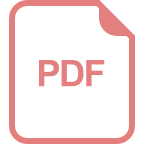
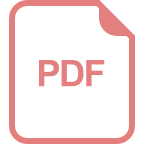
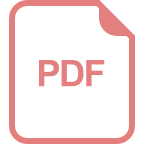
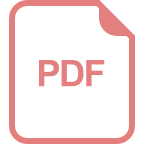
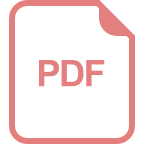
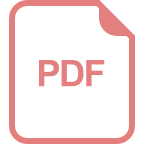
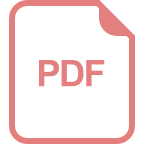
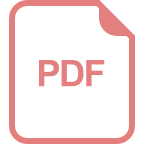
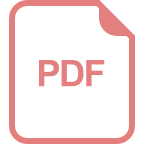
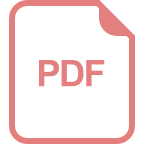
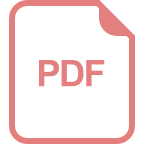
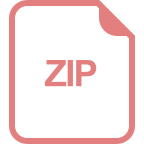
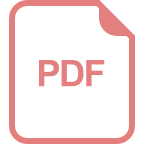
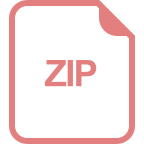

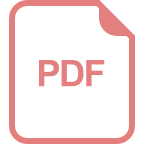