openssl RSA公钥加密代码
时间: 2023-09-28 18:12:10 浏览: 50
以下是使用 OpenSSL 库进行 RSA 公钥加密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
int rsa_public_encrypt(const unsigned char *plaintext, int plaintext_len, const char *public_key_path, unsigned char *encrypted_data) {
FILE *public_key_file = fopen(public_key_path, "rb");
if (public_key_file == NULL) {
fprintf(stderr, "Failed to open public key file.\n");
return -1;
}
RSA *rsa = RSA_new();
if (PEM_read_RSA_PUBKEY(public_key_file, &rsa, NULL, NULL) == NULL) {
fprintf(stderr, "Failed to read public key from file.\n");
fclose(public_key_file);
return -1;
}
fclose(public_key_file);
int encrypted_data_len = RSA_public_encrypt(plaintext_len, plaintext, encrypted_data, rsa, RSA_PKCS1_PADDING);
if (encrypted_data_len == -1) {
fprintf(stderr, "Failed to encrypt data.\n");
RSA_free(rsa);
return -1;
}
RSA_free(rsa);
return encrypted_data_len;
}
int main() {
const char *public_key_path = "public_key.pem"; // 替换为您的公钥文件路径
const char *plaintext = "Hello, World!";
int plaintext_len = strlen(plaintext);
unsigned char encrypted_data[256];
int encrypted_data_len = rsa_public_encrypt((unsigned char *)plaintext, plaintext_len, public_key_path, encrypted_data);
if (encrypted_data_len == -1) {
fprintf(stderr, "Failed to encrypt data.\n");
return 1;
}
printf("Encrypted data: ");
for (int i = 0; i < encrypted_data_len; i++) {
printf("%02X ", encrypted_data[i]);
}
printf("\n");
return 0;
}
```
请注意,您需要将 "public_key.pem" 替换为您实际的公钥文件路径。
这段代码首先打开公钥文件并将其读取到 RSA 结构体中。然后,它使用 `RSA_public_encrypt` 函数对明文进行加密。加密后的数据将存储在 `encrypted_data` 数组中。最后,它打印加密后的数据的十六进制表示。
请确保在编译时链接正确的 OpenSSL 库,并在运行代码之前将正确的公钥文件路径替换到代码中。
相关推荐
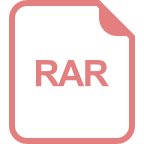
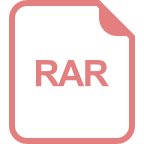














