c语言 根据年份和月份计算该月天数
时间: 2024-10-13 12:16:33 浏览: 47
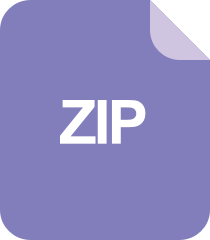
带参宏替换计算给定年份的二月天数_C语言_带参宏替换计算给定年份的二月天数_chapterbgn_

在C语言中,要根据给定的年份和月份计算该月的天数,可以使用内建库函数`mktime()`或者手动编写一段处理闰年的逻辑。这里我们先假设输入的年份和月份都是有效的,并且考虑公历的规则。
如果你想要一个简单的通用版,可以使用`ctime`库:
```c
#include <stdio.h>
#include <time.h>
int main() {
int year, month;
// 获取用户输入
printf("请输入年份和月份(格式如:2023 2):");
scanf("%d %d", &year, &month);
if(month <= 0 || month > 12) {
printf("无效的月份!\n");
return 1;
}
struct tm date = {0};
date.tm_year = year - 1900; // 转换为自1900年开始计数的年份
date.tm_mon = month - 1; // 月份从0开始计数
date.tm_mday = 1; // 初始日数设为1
// mktime()会自动处理闰年情况
time_t t = mktime(&date);
if (t == -1) {
perror("Invalid date");
return 1;
}
int days_in_month = localtime(&t)->tm_mday;
printf("给定年份和月份:%d-%d 的天数是:%d\n", year, month, days_in_month);
return 0;
}
```
然而,`mktime()`可能会导致错误,因为它依赖于系统的本地设置,对于非标准日期格式(比如某些国家的传统日历),它可能无法正确工作。如果需要更精确控制,你可以自己处理闰年的逻辑:
```c
// 自己处理闰年判断
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int get_days_in_month(int year, int month) {
switch (month) {
case 2:
return is_leap_year(year) ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
int main() {
int year, month;
// ...(同上)
int days = get_days_in_month(year, month);
printf("给定年份和月份:%d-%d 的天数是:%d\n", year, month, days);
return 0;
}
```
阅读全文
相关推荐
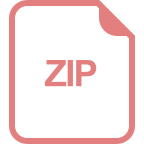
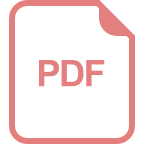



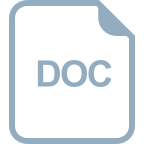











