输入年份与月份用C语言输出该月的天数
时间: 2024-09-27 09:06:58 浏览: 60
在C语言中,为了计算给定年份和月份的天数,你需要考虑闰年的规则,因为不是每个月都有30天或31天,二月通常有28天,但在闰年会有29天。以下是一个简单的函数示例,用于计算输入的年份和月份的天数:
```c
#include <stdio.h>
int is_leap_year(int year) {
if (year % 4 == 0) {
if (year % 100 == 0)
return year % 400 == 0;
else
return true;
}
return false;
}
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31; // 一般月份
case 2: // 考虑闰年的情况
return is_leap_year(year) ? 29 : 28;
case 4: case 6: case 9: case 11:
return 30;
default:
printf("Invalid month!\n");
return -1; // 表示错误的月份
}
}
int main() {
int year, month;
printf("Enter the year and month (in integer format): ");
scanf("%d %d", &year, &month);
if (month >= 1 && month <= 12) { // 检查月份是否有效
int days = days_in_month(month, year);
if (days != -1)
printf("The number of days in %d-%02d is %d.\n", year, month, days);
} else {
printf("Invalid month input!\n");
}
return 0;
}
```
在这个程序中,`is_leap_year`函数判断给定的年份是否为闰年,`days_in_month`函数则根据月份和年份返回相应的天数。用户需要输入年份和月份,然后主函数会计算并打印结果。
阅读全文
相关推荐
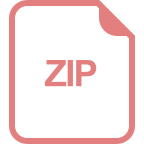
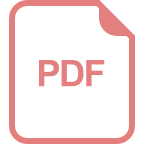
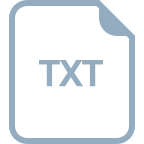















