用c语言写输入年份和月份,输出该月有多少天。
时间: 2024-10-20 10:06:34 浏览: 75
在C语言中,判断给定年份和月份的天数需要考虑闰年的规则。我们可以创建一个函数来计算特定月份的天数。下面是基本的代码示例:
```c
#include <stdio.h>
#include <stdbool.h> // 用于引入bool类型
// 判断是否为闰年
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算指定月份的天数
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12: // 全月31天
return 31;
case 4: case 6: case 9: case 11: // 全月30天
return 30;
case 2: // 考虑闰年
return is_leap_year(year) ? 29 : 28;
default:
return -1; // 无效的月份
}
}
int main() {
int year, month;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:(1-12)");
scanf("%d", &month);
if(month >= 1 && month <= 12) { // 检查月份是否有效
int days = days_in_month(month, year);
if(days != -1)
printf("该月有%d天。\n", days);
else
printf("无效的月份!\n");
} else {
printf("无效的月份!\n");
}
return 0;
}
```
这段代码首先检查输入的月份是否在1到12之间,然后根据月份和是否是闰年来确定那个月份的天数。如果是无效的月份,它将输出错误消息。
阅读全文
相关推荐
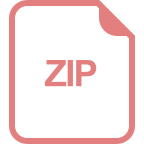
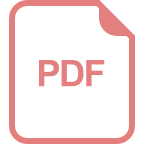
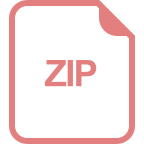















