利用该文献中的三个计算方法分别利用python编写相应的程序
时间: 2024-09-28 08:12:47 浏览: 36
针对上述文献中描述的三种阈值化算法(Algorithm 1, Algorithm 2 和 Algorithm 3),以下是基于Python语言实现这些算法的基本框架示例:
### Algorithm 1 实现
```python
import numpy as np
def entropy_based_threshold(hist):
hist = hist / np.sum(hist)
cumulative_sum = np.cumsum(hist)
def eval_function(s):
p1 = cumulative_sum[s]
p2 = 1 - p1
if p1 == 0 or p2 == 0:
return float('-inf')
max_p1 = np.max(hist[:s+1])
max_p2 = np.max(hist[s+1:])
entropy_p1 = -np.sum(hist[:s+1][hist[:s+1]>0] * np.log2(hist[:s+1][hist[:s+1]>0]))
entropy_p2 = -np.sum(hist[s+1:][hist[s+1:]>0] * np.log2(hist[s+1:][hist[s+1:]>0]))
return entropy_p1 + entropy_p2 + np.log2(max_p1) + np.log2(max_p2)
thresholds = range(len(hist))
scores = [eval_function(t) for t in thresholds]
optimal_threshold = thresholds[np.argmax(scores)]
return optimal_threshold
```
### Algorithm 2 实现
```python
def anisotropy_coefficient(hist):
cumsum_hist = np.cumsum(hist)
m = np.where(cumsum_hist >= 0.5*np.sum(hist))[0][0]
entropy_part = -np.sum(hist[:m+1] * np.log2(hist[:m+1])) if np.any(hist[:m+1]) else 0
alpha = entropy_part / np.sum(hist)
return m, alpha
def threshold_anisotropy(hist):
m, alpha = anisotropy_coefficient(hist)
if alpha < 0.5:
threshold = m
else:
threshold = m + 1
return threshold
```
### Algorithm 3 实现
```python
def new_entropy_based_threshold(hist):
hist = hist / np.sum(hist)
cumulative_sum = np.cumsum(hist)
def q_func(s):
p1 = cumulative_sum[s]
p2 = 1 - p1
if p1 == 0 or p2 == 0:
return float('-inf')
entropy_p1 = -np.sum(hist[:s+1][hist[:s+1]>0] * np.log2(hist[:s+1][hist[:s+1]>0]))
entropy_p2 = -np.sum(hist[s+1:][hist[s+1:]>0] * np.log2(hist[s+1:][hist[s+1:]>0]))
return np.log2(p1) + np.log2(p2) + entropy_p1 + entropy_p2
thresholds = range(len(hist))
scores = [q_func(t) for t in thresholds]
optimal_threshold = thresholds[np.argmax(scores)]
return optimal_threshold
```
请注意,以上代码仅提供了一个基础的框架来执行各个算法,并没有考虑所有可能的情况和边界条件处理。在实际应用时需要进一步优化和完善。同时,输入`hist`应该是一个表示灰度级别的直方图数组。
阅读全文
相关推荐









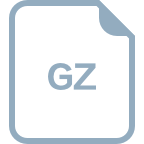








