用java算法实现尾插法创建单链表
时间: 2024-10-27 18:14:24 浏览: 16
在Java中,尾插法创建单链表主要是通过定义节点类Node,并维护一个头节点的引用。下面是一个简单的示例,展示了如何动态地在链表尾部插入新节点:
```java
// 定义链表节点
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
// 链表类
public class TailInsertionLinkedList {
private Node head;
public void insertAtTail(int value) {
// 如果链表为空,直接创建新节点并设置为头节点
if (head == null) {
head = new Node(value);
return;
}
// 否则遍历到最后一个节点
Node current = head;
while (current.next != null) {
current = current.next;
}
// 在最后一个节点后插入新节点
current.next = new Node(value);
}
// 打印链表
public void printList() {
Node temp = head;
while (temp != null) {
System.out.print(temp.data + " -> ");
temp = temp.next;
}
System.out.println("null");
}
public static void main(String[] args) {
TailInsertionLinkedList list = new TailInsertionLinkedList();
list.insertAtTail(1); // 插入第一个元素
list.insertAtTail(2); // 插入第二个元素
list.printList(); // 输出: 1 -> 2 -> null
}
}
```
这个示例中,`insertAtTail()`方法负责在链表末尾添加新节点,而`printList()`方法则是为了验证链表内容。
阅读全文
相关推荐
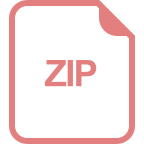
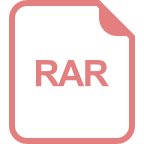
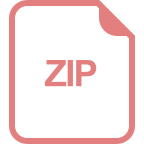
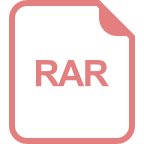
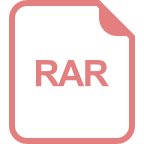
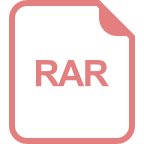
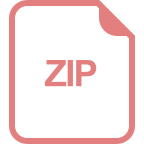
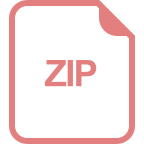
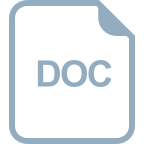
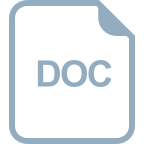
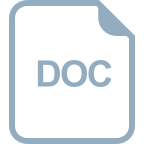
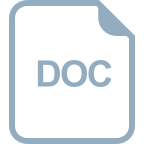
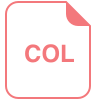
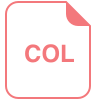
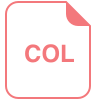
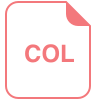
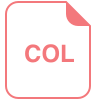
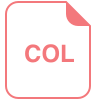
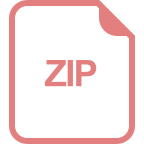