cassandra的java操作(建表、插入数据、查询)
时间: 2024-11-13 15:27:37 浏览: 3
Cassandra是一个分布式NoSQL数据库系统,特别适合处理大量高并发的数据读写。在Java中操作Cassandra通常通过Thrift API或DataStax Java Driver(现称为DataStax Java SDK)进行。以下是基本的操作步骤:
1. **建立表** (CQL, Cassandra Query Language):
```java
String keyspace = "mykeyspace";
String table = "mytable";
String createTableQuery = "CREATE KEYSPACE IF NOT EXISTS " + keyspace + " WITH replication = {'class': 'SimpleStrategy', 'replication_factor': 3};";
Session session = ... // 获取Session实例
session.execute(createTableQuery);
String createTableCql = "CREATE TABLE " + keyspace + "." + table + " (id UUID PRIMARY KEY, name text, age int);";
session.execute(createTableCql);
```
2. **插入数据**:
```java
Map<String, Object> values = new HashMap<>();
values.put("id", UUID.randomUUID());
values.put("name", "Alice");
values.put("age", 25);
BatchStatement batch = new BatchStatement(BatchType.UNLOGGED);
for (Map.Entry<String, Object> entry : values.entrySet()) {
SimpleStatement insert = new SimpleStatement(String.format("INSERT INTO %s (%s) VALUES (%s)", table, entry.getKey(), entry.getValue()));
batch.add(insert);
}
session.execute(batch);
```
3. **查询数据**:
```java
SelectStatement select = new SelectStatement(table).setConsistencyLevel(ConsistencyLevel.ONE);
select.where(eq("id", idValue));
ResultSet results = session.execute(select);
Row row = results.one(); // 获取第一条数据
System.out.println(row.getString("name")); // 输出名字
```
阅读全文
相关推荐
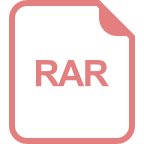
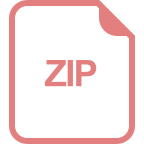
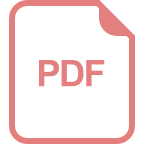

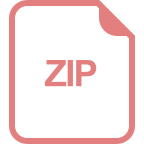
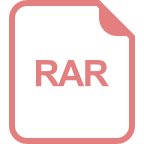
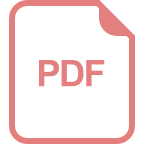
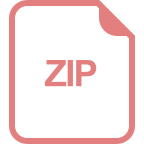
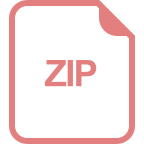
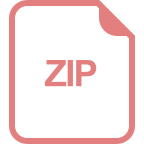
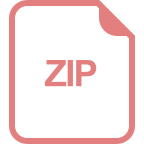
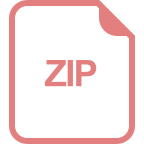
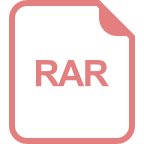
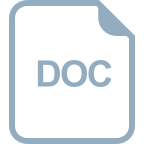
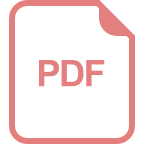
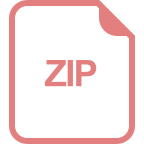