spring上传头像
时间: 2024-12-29 22:22:51 浏览: 5
### 如何在 Spring Boot 中实现用户头像上传功能
#### 创建控制器类处理文件上传请求
为了支持用户头像的上传,在 `cn.juwatech.example` 包下定义一个新的 REST 控制器来接收 HTTP POST 请求并保存接收到的图片文件到服务器端指定位置。
```java
package cn.juwatech.example.controller;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
@RestController
@RequestMapping("/api/avatar")
public class AvatarController {
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// Check if the file is empty
if (file.isEmpty()) {
return ResponseEntity.badRequest().body("Please select a file to upload.");
}
// Get file details
String fileName = file.getOriginalFilename();
// Save the file locally or in database as per requirement.
byte[] bytes = file.getBytes();
// Here you can add logic to save these bytes into your DB
return ResponseEntity.ok("You successfully uploaded " + fileName);
} catch (Exception e) {
return ResponseEntity.status(500).body(e.getMessage());
}
}
}
```
此代码片段展示了一个基本的文件上传接口[^2]。当客户端发送带有表单数据 multipart/form-data 类型的内容时,该方法会解析出其中名为 'file' 的字段作为待上传的文件对象,并对其进行初步验证和存储操作。
#### 前端页面设计用于触发上传动作
对于前端部分,则需构建一个 HTML 表单允许用户选择本地计算机上的图像文件并通过 AJAX 或者直接提交的方式调用上述 API 接口完成实际的数据传输过程[^3]。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Upload Profile Picture</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h2>Select profile picture:</h2>
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" name="file"/>
<button type="submit">Submit</button>
</form>
<div id="message"></div>
<script>
$(document).ready(function() {
$('#uploadForm').on('submit', function(event){
event.preventDefault();
$.ajax({
url : '/api/avatar/upload',
type: 'POST',
data: new FormData(this),
processData: false,
contentType: false,
success : function(result) {
$('#message').text(result);
},
error : function(errorThrown){
console.log(errorThrown);
}
});
});
});
</script>
</body>
</html>
```
这段脚本实现了通过 jQuery 发送异步请求的功能,它会在点击按钮后阻止默认行为并将整个表单序列化为 FormData 对象传递给后台服务进行进一步处理。
阅读全文
相关推荐
















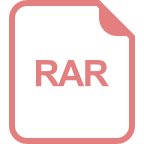

