用java举例 comparator用法
时间: 2023-09-05 13:11:21 浏览: 132
Comparator是Java中用于比较对象的接口,它可以用于定义对象的排序规则。以下是一个使用Comparator的简单例子:
假设我们有一个名为Student的类,其中包含学生的姓名和年龄属性。我们想按照学生的年龄对它们进行排序。
首先,我们需要创建一个实现Comparator接口的类,该类将定义比较规则。例如,我们可以创建一个名为AgeComparator的类:
```java
import java.util.Comparator;
public class AgeComparator implements Comparator<Student> {
@Override
public int compare(Student s1, Student s2) {
if (s1.getAge() < s2.getAge()) {
return -1; // s1的年龄小于s2的年龄
} else if (s1.getAge() > s2.getAge()) {
return 1; // s1的年龄大于s2的年龄
} else {
return 0; // 年龄相等
}
}
}
```
然后,在我们的主程序中,我们可以使用这个AgeComparator来对Student对象进行排序:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 20));
students.add(new Student("Bob", 18));
students.add(new Student("Charlie", 22));
// 使用AgeComparator对学生列表进行排序
Collections.sort(students, new AgeComparator());
// 打印排序后的学生列表
for (Student student : students) {
System.out.println(student.getName() + " - " + student.getAge());
}
}
}
```
在上面的代码中,我们创建了一个包含三个学生对象的列表,并使用`Collections.sort()`方法对其进行排序。通过传入AgeComparator对象作为排序规则,我们可以实现根据学生的年龄进行排序。最后,我们打印排序后的学生列表。
阅读全文
相关推荐














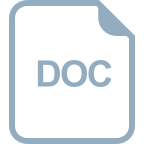


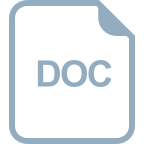
