three.js鼠标移到模型上显示文字标签
时间: 2024-09-13 17:02:39 浏览: 71
在three.js中,要实现鼠标移动到模型上显示文字标签,通常需要使用场景中的对象拾取机制,配合渲染文字标签的UI元素。这里有一些基本的步骤:
1. **监听鼠标事件**:首先需要为场景添加鼠标事件监听器,如`mousemove`事件,当鼠标移动到场景上时,根据鼠标的屏幕坐标找到最接近的场景中的对象。
2. **对象拾取**:使用射线投射(Raycasting)技术来检测鼠标位置下最接近的物体。射线从摄像机发射,穿过鼠标所在的屏幕位置,然后与场景中的对象进行相交检测。
3. **显示文字标签**:一旦检测到对象,可以在该对象附近渲染一个HTML元素,用来显示文字标签。可以通过创建一个div元素,并使用CSS样式来定位它在3D空间的正确位置。
4. **更新和隐藏标签**:当鼠标移动到其他位置时,需要更新射线投射的检测结果,并相应地更新标签的位置或者隐藏标签。
下面是一个简单的代码示例,展示了如何结合上述步骤:
```javascript
// 初始化场景、相机、渲染器等...
// 监听鼠标移动事件
document.addEventListener('mousemove', onDocumentMouseMove, false);
var mouse = new THREE.Vector2(); // 用于存储鼠标位置
function onDocumentMouseMove(event) {
// 将鼠标位置转换为归一化的设备坐标
mouse.x = (event.clientX / window.innerWidth) * 2 - 1;
mouse.y = - (event.clientY / window.innerHeight) * 2 + 1;
// 使用射线投射检测最接近的对象
raycaster.setFromCamera(mouse, camera);
var intersects = raycaster.intersectObjects(scene.children);
if (intersects.length > 0) {
// 如果有对象被选中,显示标签
showLabel(intersects[0].object);
} else {
// 如果没有对象被选中,隐藏标签
hideLabel();
}
}
function showLabel(selectedObject) {
// 创建标签
var label = document.createElement('div');
label.style.position = 'absolute';
label.style.background = 'white';
label.style.border = '1px solid black';
label.style.padding = '5px';
label.style.zIndex = 100;
document.body.appendChild(label);
// 设置标签位置和内容
var labelPosition = new THREE.Vector3();
var projector = new THREE.Projector();
projector.projectVector(selectedObject.position, camera);
label.style.left = (projector.projectVector(selectedObject.position, camera).x * window.innerWidth / 2) + 'px';
label.style.top = (projector.projectVector(selectedObject.position, camera).y * window.innerHeight / -2) + 'px';
label.textContent = '这是标签内容'; // 可以根据需要显示不同的内容
}
function hideLabel() {
// 隐藏标签
var label = document.getElementById('label');
if (label) {
label.remove();
}
}
// 渲染循环中调用渲染器...
```
上述代码提供了一个基本的框架,你可能需要根据实际的应用需求对`showLabel`函数进行更多的定制,比如动态设置标签的样式、位置或者绑定更复杂的内容。
阅读全文
相关推荐
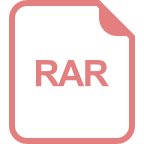
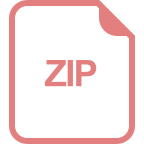
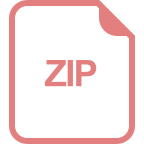
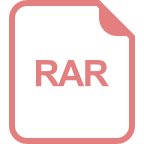
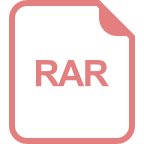
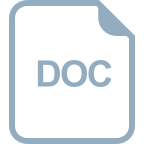
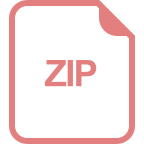
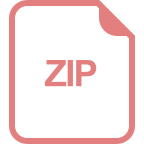
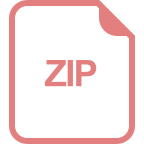
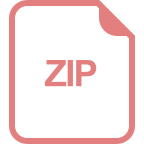
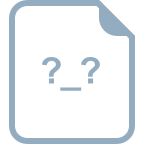
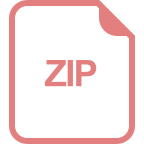
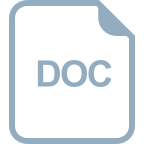
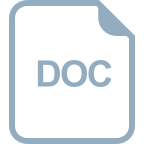
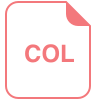
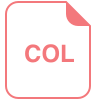
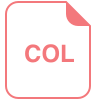
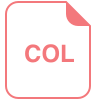
