帮我用python 写一段贪吃蛇代码
时间: 2024-06-19 09:00:49 浏览: 105
以下是一个简单的Python贪吃蛇游戏代码的示例[^2]:
```python
import pygame
import random
# 游戏窗口大小
WIDTH = 800
HEIGHT = 600
# 贪吃蛇方块大小
BLOCK_SIZE = 20
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 初始化pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇游戏")
# 贪吃蛇类
class Snake:
def __init__(self):
self.body = [(WIDTH // 2, HEIGHT // 2)]
self.direction = random.choice(["UP", "DOWN", "LEFT", "RIGHT"])
def move(self):
x, y = self.body[0]
if self.direction == "UP":
y -= BLOCK_SIZE
elif self.direction == "DOWN":
y += BLOCK_SIZE
elif self.direction == "LEFT":
x -= BLOCK_SIZE
elif self.direction == "RIGHT":
x += BLOCK_SIZE
self.body.insert(0, (x, y))
self.body.pop()
def change_direction(self, new_direction):
if new_direction == "UP" and self.direction != "DOWN":
self.direction = new_direction
elif new_direction == "DOWN" and self.direction != "UP":
self.direction = new_direction
elif new_direction == "LEFT" and self.direction != "RIGHT":
self.direction = new_direction
elif new_direction == "RIGHT" and self.direction != "LEFT":
self.direction = new_direction
def draw(self):
for x, y in self.body:
pygame.draw.rect(window, GREEN, (x, y, BLOCK_SIZE, BLOCK_SIZE))
# 食物类
class Food:
def __init__(self):
self.x = random.randint(0, WIDTH - BLOCK_SIZE) // BLOCK_SIZE * BLOCK_SIZE
self.y = random.randint(0, HEIGHT - BLOCK_SIZE) // BLOCK_SIZE * BLOCK_SIZE
def draw(self):
pygame.draw.rect(window, RED, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE))
# 创建贪吃蛇和食物对象
snake = Snake()
food = Food()
# 游戏主循环
running = True
clock = pygame.time.Clock()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.change_direction("UP")
elif event.key == pygame.K_DOWN:
snake.change_direction("DOWN")
elif event.key == pygame.K_LEFT:
snake.change_direction("LEFT")
elif event.key == pygame.K_RIGHT:
snake.change_direction("RIGHT")
snake.move()
# 判断是否吃到食物
if snake.body[0] == (food.x, food.y):
snake.body.append((food.x, food.y))
food = Food()
# 判断是否撞墙或撞到自己
if snake.body[0][0] < 0 or snake.body[0][0] >= WIDTH or snake.body[0][1] < 0 or snake.body[0][1] >= HEIGHT:
running = False
for i in range(1, len(snake.body)):
if snake.body[0] == snake.body[i]:
running = False
# 绘制游戏界面
window.fill(BLACK)
snake.draw()
food.draw()
pygame.display.flip()
# 控制游戏帧率
clock.tick(10)
pygame.quit()
```
阅读全文
相关推荐
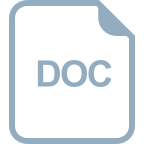
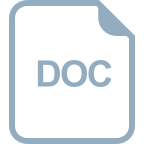
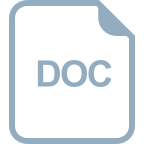








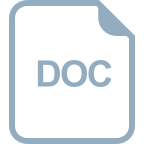
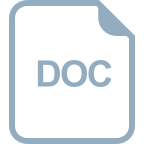
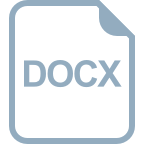
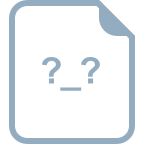
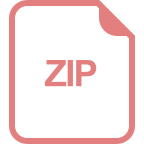