matlab多条直线分割多边形
时间: 2024-03-28 20:34:34 浏览: 114
在Matlab中,可以使用多种方法将多条直线分割多边形。以下是两种常用的方法:
1. 使用交点法[^1]:
- 首先,定义多边形的顶点坐标和直线的参数方程。
- 然后,使用循环遍历每条直线,计算直线与多边形的交点。
- 最后,根据交点的位置将多边形分割成多个子多边形。
示例代码如下:
```matlab
% 定义多边形的顶点坐标
polygon = [1, 1; 2, 3; 4, 2; 3, 1];
% 定义直线的参数方程
line_eq = @(t) [t, 0.5*t+2];
% 初始化分割后的多边形
polygons = {polygon};
% 遍历每条直线
for t = 0:0.1:1
% 计算直线与多边形的交点
intersection_points = [];
for i = 1:size(polygon, 1)
p1 = polygon(i, :);
p2 = polygon(mod(i, size(polygon, 1)) + 1, :);
[x, y] = lineintersect(p1, p2, line_eq(t), line_eq(t+0.1));
if ~isempty(x) && ~isempty(y)
intersection_points = [intersection_points; x, y];
end
end
% 根据交点的位置分割多边形
new_polygons = {};
for i = 1:length(polygons)
polygon = polygons{i};
inside_points = inpolygon(intersection_points(:, 1), intersection_points(:, 2), polygon(:, 1), polygon(:, 2));
if any(inside_points)
inside_points = [inside_points; inside_points(1)];
inside_indices = find(inside_points);
for j = 1:length(inside_indices)-1
new_polygon = polygon(inside_indices(j):inside_indices(j+1), :);
new_polygons = [new_polygons, {new_polygon}];
end
else
new_polygons = [new_polygons, {polygon}];
end
end
polygons = new_polygons;
end
% 输出分割后的多边形
for i = 1:length(polygons)
disp(polygons{i});
end
```
2. 使用边界交点法:
- 首先,定义多边形的顶点坐标和直线的参数方程。
- 然后,计算直线与多边形的边界的交点。
- 最后,根据交点的位置将多边形分割成多个子多边形。
示例代码如下:
```matlab
% 定义多边形的顶点坐标
polygon = [1, 1; 2, 3; 4, 2; 3, 1];
% 定义直线的参数方程
line_eq = @(t) [t, 0.5*t+2];
% 初始化分割后的多边形
polygons = {polygon};
% 计算直线与多边形的边界的交点
intersection_points = [];
for i = 1:size(polygon, 1)
p1 = polygon(i, :);
p2 = polygon(mod(i, size(polygon, 1)) + 1, :);
[x, y] = lineintersect(p1, p2, line_eq(0), line_eq(1));
if ~isempty(x) && ~isempty(y)
intersection_points = [intersection_points; x, y];
end
end
% 根据交点的位置分割多边形
new_polygons = {};
for i = 1:length(polygons)
polygon = polygons{i};
inside_points = inpolygon(intersection_points(:, 1), intersection_points(:, 2), polygon(:, 1), polygon(:, 2));
if any(inside_points)
inside_points = [inside_points; inside_points(1)];
inside_indices = find(inside_points);
for j = 1:length(inside_indices)-1
new_polygon = polygon(inside_indices(j):inside_indices(j+1), :);
new_polygons = [new_polygons, {new_polygon}];
end
else
new_polygons = [new_polygons, {polygon}];
end
end
% 输出分割后的多边形
for i = 1:length(polygons)
disp(polygons{i});
end
```
阅读全文
相关推荐




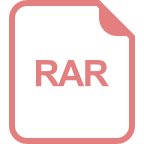











