如何将Qt中的16进制字符串转换为整数类型(int)?
时间: 2024-10-25 13:13:57 浏览: 67
在Qt中,你可以使用`QChar::fromHex()`函数以及`std::stoi()`或者`QLatin1String().toInt()`方法将十六进制字符串转换为整数类型(int)。下面是一个简单的示例:
```cpp
#include <QString>
#include <QChar>
#include <iostream>
// 将十六进制字符串转换为整数
int hexStringToInteger(const QString& hexStr) {
// 使用QChar::fromHex()获取每个字符对应的十进制值
QByteArray bytes;
for (const QChar ch : hexStr) {
if (ch.isDigit()) {
bytes.append(ch.unicode());
} else if (ch == QLatin1Char('a') || ch == QLatin1Char('A')) { // 对于小写字母'a'到'f'
bytes.append(ch.unicode() - 0x30 + 10);
} else if (ch == QLatin1Char('b') || ch == QLatin1Char('B')) { // 对于大写字母'b'到'f'
bytes.append(ch.unicode() - 0x41 + 10);
} else {
std::cerr << "Invalid character in hexadecimal string" << std::endl;
return -1; // 如果遇到无效字符,返回错误
}
}
// 将字节数组转换为整数
int value = static_cast<int>(bytes.join("").toUtf8().toInt(16)); // 第二个参数指定基数为16
return value;
}
int main() {
QString hexStr = "FF"; // 一个例子十六进制字符串
int num = hexStringToInteger(hexStr);
if (num != -1) {
std::cout << "The integer representation is: " << num << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
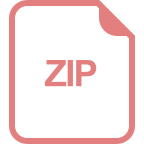
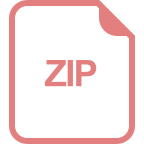
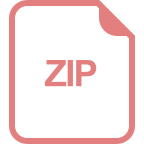















