char+aChar:chars
时间: 2024-03-31 08:28:10 浏览: 110
根据提供的引用内容,可以得知char和aChar都是字符类型,而chars则是一个不确定的变量名,无法确定其具体含义。如果你想要将两个字符拼接成一个字符串,可以使用字符串流stringstream或者使用字符串拼接符号+来实现。具体方法如下所示:
1.使用stringstream:
```c++
#include <iostream>
#include <sstream>
using namespace std;
int main() {
char char1 = 'a';
char char2 = 'b';
stringstream ss;
ss << char1 << char2;
string result = ss.str();
cout << result << endl; // 输出:ab
return 0;
}
```
2.使用字符串拼接符号+:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
char char1 = 'a';
char char2 = 'b';
string result = "";
result += char1;
result += char2;
cout << result << endl; // 输出:ab
return 0;
}
```
相关问题
for (char aChar : chars)
This is a for-each loop in Java that iterates over every character (aChar) in an array or collection of characters (chars).
The loop syntax can be read as follows: "For each character aChar in the array or collection chars, do the following code block".
The loop will automatically iterate through each element in the array or collection without requiring an index variable to be declared or incremented.
This type of loop is useful when you want to perform a task on every element in an array or collection, without having to manually manage the index values.
怎么添加char字符到std::string中
可以使用 std::string 的成员函数 `push_back()` 或 `append()` 来添加 char 字符到 std::string 中。
1. 使用 `push_back()` 函数:
```cpp
std::string str;
char ch = 'a';
str.push_back(ch);
```
2. 使用 `append()` 函数:
```cpp
std::string str;
char ch = 'a';
str.append(1, ch);
```
以上两种方法都可以将字符 'a' 添加到字符串 str 的末尾。如果需要添加多个字符,可以使用 `append()` 函数,如下所示:
```cpp
std::string str;
const char* chars = "hello";
str.append(chars, 5); // 添加前 5 个字符
```
以上代码将字符串 "hello" 的前 5 个字符添加到字符串 str 的末尾。
另外,还可以使用 `+` 运算符将字符添加到字符串的末尾,例如:
```cpp
std::string str = "hello";
char ch = '!';
str += ch; // 添加字符 '!'
```
以上代码将字符 '!' 添加到字符串 str 的末尾。
阅读全文
相关推荐
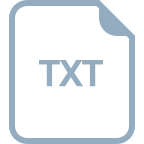
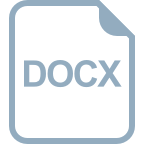
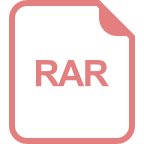













