Given a string, return a string where for every char in the original, there are two chars.
时间: 2024-02-02 11:04:34 浏览: 28
Sure! Here's a Python code snippet that doubles every character in a given string:
``` python
def double_char(string):
doubled_string = ''
for char in string:
doubled_string += char * 2
return doubled_string
```
You can call this function with a string as its argument and it will return a new string where each character in the original string is repeated twice. For example:
``` python
>>> double_char('hello')
'hheelllloo'
>>> double_char('1234')
'11223344'
>>> double_char('!')
'!!'
```
Let me know if you have any other questions!
相关问题
Given a string, return the length of the largest "block" in the string. A block is a run of adjacent chars that are the same.
Sure, I can help you with that. Here's a Python function that takes a string as input and returns the length of the largest block:
```python
def largest_block(s):
max_block_len = 0
block_len = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
block_len += 1
else:
max_block_len = max(max_block_len, block_len)
block_len = 1
return max(max_block_len, block_len)
```
Here's how you can use this function:
```python
s = "abbcccdddd"
print(largest_block(s)) # Output: 4
```
In this example, the largest block in the string `s` is `"dddd"`, which has a length of 4.
Given a string s, find the length of the longest substring without repeating characters.
To find the length of the longest substring without repeating characters, we can use the sliding window approach. Here's the algorithm:
1. Initialize a set to store the unique characters in the current window, and two pointers left and right to mark the current window.
2. Initialize a variable max_len to 0 to store the length of the longest substring without repeating characters.
3. While the right pointer is less than the length of the string s:
- If the character at s[right] is not in the set, add it to the set and move the right pointer to the right.
- Else, remove the character at s[left] from the set and move the left pointer to the right.
4. Update the value of max_len with the maximum of its current value and the size of the set.
5. Return max_len.
Here's the Python code for the algorithm:
```
def longest_substring(s):
unique_chars = set()
left = 0
max_len = 0
for right in range(len(s)):
while s[right] in unique_chars:
unique_chars.remove(s[left])
left += 1
unique_chars.add(s[right])
max_len = max(max_len, len(unique_chars))
return max_len
```
Example usage:
```
s = "abcabcbb"
print(longest_substring(s)) # Output: 3 (for "abc")
```
相关推荐
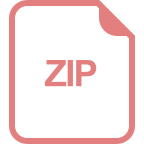












