用java解决问题。 Given a string, return the sum of the digits 0-9 that appear in the string, ignoring all other characters. Return 0 if there are no digits in the string. (Note: Character.isDigit(char) tests if a char is one of the chars '0', '1', .. '9'. Integer.parseInt(string) converts a string to an int.)
时间: 2023-06-25 08:06:40 浏览: 71
可以使用Java中的正则表达式来实现该问题。具体实现步骤如下:
1.使用正则表达式匹配字符串中的所有数字,可以使用`\\d`表示数字。
2.将匹配到的数字字符串转换为整数类型并累加。
3.如果没有匹配到数字,则返回0。
下面是代码实现:
```java
public int sumDigits(String str) {
int sum = 0;
Pattern pattern = Pattern.compile("\\d"); // 正则表达式,匹配数字
Matcher matcher = pattern.matcher(str);
while (matcher.find()) { // 查找匹配到的数字,并累加
sum += Integer.parseInt(matcher.group());
}
return sum;
}
```
使用示例:
```java
String str = "abc123def456";
int sum = sumDigits(str);
System.out.println(sum); // 输出 21,即 1+2+3+4+5+6
```
相关问题
write a Java program called SumOfDigits which reads an integer between 0 and 1000 and adds all the digits in the integer.
Sure, here's the Java program to solve your problem:
```
import java.util.Scanner;
public class SumOfDigits {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer between 0 and 1000: ");
int num = input.nextInt();
int sum = 0;
while (num > 0) {
sum += num % 10;
num /= 10;
}
System.out.println("The sum of the digits is " + sum);
}
}
```
This program prompts the user to enter an integer between 0 and 1000 and then uses a while loop to add up all the digits in the integer. The program then prints out the sum of the digits.
use C program Write a method that computes the sum of the digits in an integer. Use the following method header: int sumDigits(int n)
Sure, here's a C program that implements the `sumDigits` method as described:
```c
#include <stdio.h>
int sumDigits(int n);
int main() {
int n;
printf("Enter an integer: ");
scanf("%d", &n);
printf("The sum of digits in %d is %d\n", n, sumDigits(n));
return 0;
}
int sumDigits(int n) {
int sum = 0;
while (n != 0) {
int digit = n % 10;
sum += digit;
n /= 10;
}
return sum;
}
```
The `sumDigits` method takes an integer `n` as input, and returns the sum of the digits in `n`. The main function prompts the user to input an integer, calls `sumDigits` to compute the sum of its digits, and prints the result. The `sumDigits` method uses a while loop to iterate over each digit in `n`, adding it to a running total until all digits have been processed.
相关推荐
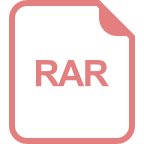
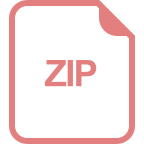












