用c++解决For example, if you want to exchange 100 US Dollars into Russian Rubles at the exchange point, where the exchange rate is 29.75, and the commission is 0.39 you will get (100 - 0.39) * 29.75 = 2963.3975RUR. You surely know that there are N different currencies you can deal with in our city. Let us assign unique integer number from 1 to N to each currency. Then each exchange point can be described with 6 numbers: integer A and B - numbers of currencies it exchanges, and real RAB, CAB, RBA and CBA - exchange rates and commissions when exchanging A to B and B to A respectively. Nick has some money in currency S and wonders if he can somehow, after some exchange operations, increase his capital. Of course, he wants to have his money in currency S in the end. Help him to answer this difficult question. Nick must always have non-negative sum of money while making his operations. Input The first line contains four numbers: N - the number of currencies, M - the number of exchange points, S - the number of currency Nick has and V - the quantity of currency units he has. The following M lines contain 6 numbers each - the description of the corresponding exchange point - in specified above order. Numbers are separated by one or more spaces. 1 ≤ S ≤ N ≤ 100, 1 ≤ M ≤ 100, V is real number, 0 ≤ V ≤ 103. For each point exchange rates and commissions are real, given with at most two digits after the decimal point, 10-2 ≤ rate ≤ 102, 0 ≤ commission ≤ 102. Let us call some sequence of the exchange operations simple if no exchange point is used more than once in this sequence. You may assume that ratio of the numeric values of the sums at the end and at the beginning of any simple sequence of the exchange operations will be less than 104. Output If Nick can increase his wealth, output YES, in other case output NO.
时间: 2024-02-15 10:27:38 浏览: 82
以下是用 C++ 解决该问题的代码:
```
#include <iostream>
#include <vector>
#include <cstring>
#include <cmath>
using namespace std;
const double eps = 1e-6;
const double INF = 1e9;
struct Edge {
int from, to;
double rate, commission;
};
vector<Edge> edges;
double dis[105];
int n, m, s;
double v;
bool bellman_ford() {
memset(dis, 0, sizeof(dis));
dis[s] = v;
for (int i = 1; i <= n; i++) {
for (int j = 0; j < edges.size(); j++) {
Edge e = edges[j];
if (dis[e.from] > eps && dis[e.from] * e.rate - e.commission > dis[e.to]) {
dis[e.to] = dis[e.from] * e.rate - e.commission;
if (i == n) return true;
}
}
}
return false;
}
int main() {
cin >> n >> m >> s >> v;
for (int i = 1; i <= m; i++) {
int a, b;
double rab, cab, rba, cba;
cin >> a >> b >> rab >> cab >> rba >> cba;
edges.push_back({a, b, rab, cab});
edges.push_back({b, a, rba, cba});
}
if (bellman_ford()) cout << "YES" << endl;
else cout << "NO" << endl;
return 0;
}
```
该问题可以转化为一个图论问题。将每个货币看做一个点,每个兑换点看做一条有向边,边权为兑换汇率,边上还有一个手续费。然后我们需要判断是否存在一条简单路径,使得起点为 S,终点为 S,路径上的货币价值不断增加。
我们可以使用 Bellman-Ford 算法来求解该问题。由于存在手续费,每次更新时需要判断是否能够使货币价值增加,并且需要注意精度问题。
具体实现见代码。
阅读全文
相关推荐
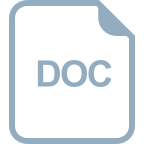
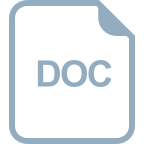
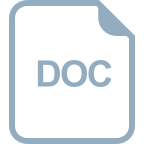

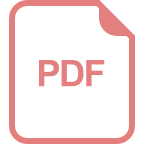
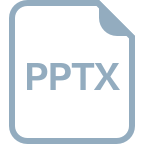
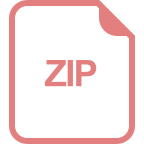
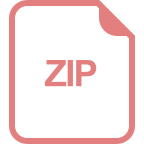
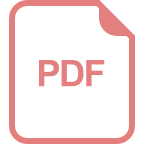
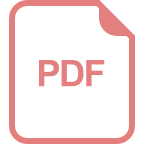
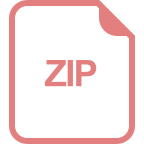
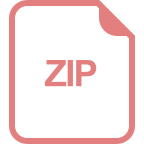
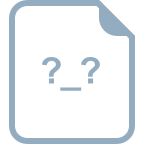
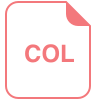
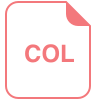



