Python基础指令
时间: 2024-09-11 13:14:26 浏览: 44
Python是一种高级编程语言,它强调代码的可读性和简洁的语法(尤其是使用空格缩进来表示代码块,而不是使用大括号或关键字)。这里介绍一些Python的基础指令:
1. 打印输出:使用`print()`函数来在控制台输出信息。
```python
print("Hello, World!")
```
2. 变量声明:在Python中,不需要显式声明变量类型,直接赋值即可创建变量。
```python
x = 10
y = "Python"
```
3. 基本数据类型:Python有几种内置的数据类型,包括字符串(str)、整型(int)、浮点型(float)、布尔型(bool)和列表(list)等。
```python
a = "字符串"
b = 123
c = 12.34
d = True
e = [1, 2, 3]
```
4. 控制流程:使用`if`语句进行条件判断,使用`for`和`while`进行循环。
```python
if a > b:
print("a 大于 b")
for i in range(5):
print(i)
j = 0
while j < 5:
print(j)
j += 1
```
5. 函数定义:使用`def`关键字来定义函数。
```python
def my_function(param1, param2):
return param1 + param2
```
6. 模块导入:Python使用`import`语句来导入模块。
```python
import math
result = math.sqrt(16)
```
7. 异常处理:使用`try`和`except`语句来处理程序运行时可能出现的错误。
```python
try:
num = int(input("请输入一个整数: "))
except ValueError:
print("输入错误")
```
8. 文件操作:使用`open()`函数来打开文件,并进行读写等操作。
```python
file = open("example.txt", "r")
content = file.read()
file.close()
```
阅读全文
相关推荐
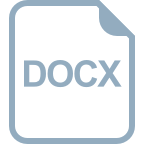
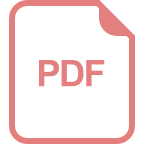
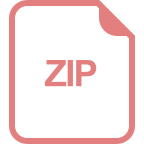
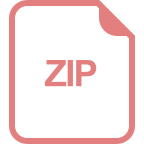
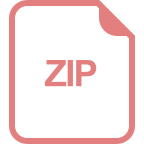
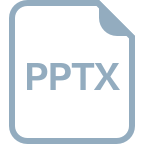
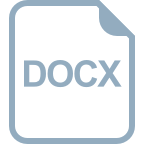
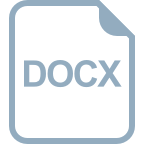
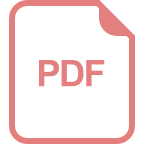
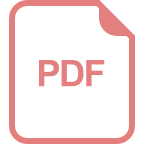
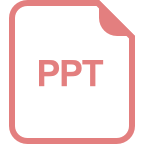
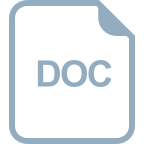

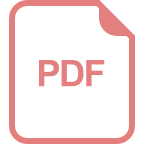
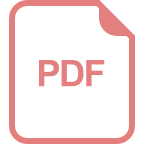
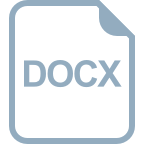